Wednesday, June 26, 2013
I'm a Customer
Blog Entry #293
Having dispatched the running order part of the "So, You Want A Shopping Cart, Huh?" order.html page in the last couple of entries, we turn our attention now to the customer/shipping part of the page.
The
tables[3]
table Just below the item detail section of the order.html page is a two-row, two-cell table whose upper cell holds a formatted Comments & Additional Information Can be written in the box below label and whose lower cell holds a textarea field for inputting said comments and additional information.
<table border="0" width="400"><tr><td align="center" colspan="6" bgcolor="#ff9999">
<b>Comments & Additional Information<br><font color="#ffff00">Can be written in the box below</font><br></b>
</td></tr><tr><td colspan="6">
<center><textarea name="comments" rows="10" cols="40"></textarea></center>
</td></tr></table>
Do the
colspan="6"
attributes serve any purpose? Nope: chuck 'em. Why is the textarea field centered with a center element vis-à-vis an align="center"
td attribute? Don't know. Can and should the tables[3]
table be recast as a div? Yep:#commentDiv { text-align: center; width: 400px; }
#commentLabel { background-color: #ff9999; font-weight: bold; display: block; width: 400px; }
#commentSpan { color: yellow; }
...
<div id="commentDiv">
<label id="commentLabel" for="commentValue">Comments & Additional Information<br>
<span id="commentSpan">Can be written in the box below</span></label>
<textarea id="commentValue" name="comments" rows="10" cols="40"></textarea>
</div>
The
tables[4]
tableThe user inputs her/his contact information into the fields of a Customer Information / Details table just below the
tables[3]
table. The tables[4]
table comprises twelve rows and twenty-four cells; rather than burden you with its code, why don't I just show you a picture?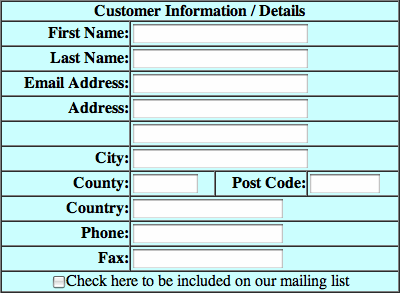
(I've given the table a
border="1"
as a means of sorting out its colspan situation - vide infra.)American users will want to replace the County: field with a State: field. BTW, the Royal Mail doesn't make use of postal county data anymore, so our friends in the UK can throw out the County: field altogether.
The absence of a label for the fifth
tables[4]
field may confuse some users; this field is meant to be an address field, i.e., it is for continuing the value of the preceding Address: field or for holding a second address, so we should really give it an Address #2: label, wouldn't you say?Most of the
tables[4]
fields are 'required': the user must provide values for all of the fields except the Address #2:, Phone:, and Fax: fields in order to submit the order form; however, this information is not conveyed to the user.Note that the eighth
tables[4]
row contains four cells. The first tables[4]
row contains one cell whose colspan is set to 5 but should be set to 4; ditto for the twelfth tables[4]
row. For the remaining rows, the field cell colspan is correctly set to 3. All of the left-hand label cells have a colspan="2"
setting, which can be removed as these cells do not span two table columns.Other presentational notes:
(1) The
tables[4]
table itself has a bgcolor="#00ffff"
attribute, which is overridden by the bgcolor="#ccffff"
attribute(s) given to each cell.(2) All of the labels are bolded and right-justified.
Lastly, the first-row Customer Information / Details header can and probably should be marked up as a caption element:
#userCaption { background-color: #ccffff; font-weight: bold; }
...
<table border="" cellpadding="0" cellspacing="0" width="400">
<caption id="userCaption">Customer Information / Details</caption> ...
The
tables[5]
tableThe recipient's contact details are entered into the fields of a Shipping Address: table just below the
tables[4]
table.
Most of the above
tables[4]
table commentary also applies to the tables[5]
table: the fourth row needs an Address #2: label, all fields except the Address #2: and Phone: fields are required but are not marked as such, etc.Upon
<caption>
-izing the Shipping Address: header, I find that the browsers on my computer will not let me put the caption and the Same as above control in the same line box; in this case the latter must be placed in its own <tr>
.Checking the Same as above checkbox
<td align="center" colspan="3" bgcolor="#ccffff">
<input type="checkbox" name="same_flag" onclick="copyToShipping( );">
<font size="2" color="#ff0000"><blink>Same as above</blink></font></td>
calls the order.html copyToShipping( ) function, which copies the
tables[4]
table's name, snail-mail address, and phone data to the tables[5]
table.function copyToShipping( ) {
if (document.order.same_flag.checked) {
document.order.ship_name.value = document.order.name_first.value + " " + document.order.name_last.value;
document.order.ship_address1.value = document.order.address1.value;
document.order.ship_address2.value = document.order.address2.value;
document.order.ship_city.value = document.order.city.value;
document.order.ship_state.value = document.order.state.value;
document.order.ship_zip.value = document.order.zip.value;
document.order.ship_country.value = document.order.country.value;
document.order.ship_phone.value = document.order.phone.value; } }
Interestingly, the Same as above string is wrapped in a blink element, which
makes text blink on and off,quoting Netscape. The blink element, its text-decoration:blink; CSS equivalent, and its stringObject.blink( ); JavaScript equivalent are today supported by Mozilla's browsers (Firefox, Camino, Netscape 9) and Opera but not by IE, Google Chrome, and Safari. Wikipedia's blink element entry notes that blinking text is an 'accessibility no-no', so the blink markup should probably be removed.
Changing the value of any of the
tables[5]
fields calls the order.html disableSameFlag( ) function, which sets the checked status of the same_flag checkbox to false irrespective of whether the checkbox is checked or not. function disableSameFlag( ) { document.order.same_flag.checked = false; }
...
<input type="text" size="30" maxlength="50" name="ship_name" onchange="disableSameFlag( );">
Finally, the
tables[5]
table has an empty ninth row<tr><td> </td></tr>
that should be thrown out.
Functional compaction
If we give the
tables[4]
table an id="userTable"
and the tables[5]
table an id="shippingTable"
, then the copyToShipping( ) functionality can be replaced with:var userInputs = document.getElementById("userTable").getElementsByTagName("input");
var shippingInputs = document.getElementById("shippingTable").getElementsByTagName("input");
shippingInputs[0].onclick = function ( ) {
shippingInputs[1].value = userInputs[0].value + " " + userInputs[1].value;
for (i = 2; i < shippingInputs.length; i++) shippingInputs[i].value = userInputs[i + 1].value; }
With the shippingInputs element collection in hand, the disableSameFlag( ) functionality can be recast as:
for (i = 1; i < shippingInputs.length; i++)
shippingInputs[i].onchange = function ( ) { document.order.same_flag.checked = false; }
The
tables[6]
tableThis table contains a Choose Order Method: header in its first row and a Phone Call: control in its second row

and at first glance seems pointless - not much of a choice, is it? why don't we just replace it with a phone number for placing an order? - although we'll see later that Joe does put the control to use, namely, he conditions the submission of the order form in part on the radio button's checked status.
The
tables[6]
table has a third row whose only 'content' is an anchor element start-tag:<tr><td align="center" colspan="5" bgcolor="#ccffff"><a href="options.htm" target="navigate"></td></tr>
The
<a href="options.htm" target="navigate">
tag is a remnant of the Learn about the different ordering methods link in the Choose Order Method: table at Gordon's order.htm page. I'd say we can safely excise the <a href="options.htm" target="navigate">
row from Joe's table, wouldn't you?Even if we hold onto the Phone Call: control, the
tables[6]
table should be converted to a div:#ordermethodDiv { background-color: #ccffff; font-size: large; font-weight: bold; text-align: center; width: 400px; }
#ordermethodLabel { font-size: small; }
...
<div id="ordermethodDiv">Choose Order Method:<br>
<label id="ordermethodLabel">Phone Call: <input type="radio" name="order_type" value="phone"></label></div>
The
tables[7]
tableHere's what it should look like:

Here's how we should code it:
#intlshippingDiv { background-color: #ccffff; font-weight: bold; text-align: center; width: 400px; }
#intlshippingSpan { font-size: large; }
...
<div id="intlshippingDiv"><span id="intlshippingSpan">International Shipping:</span><br>
Prices cover shipment worldwide</div>
'Nuff said.
Perhaps the Customer Information / Details and Shipping Address: tables should also be converted to divs, but these tables at least somewhat resemble grids of data, and therefore I am inclined to let their table architectures be.
One more point before we submit the order form:
If we're going to remove the credit card stuff (the On-line / Credit Card: option in the Choose Order Method: table and the Credit Card Info: table) from the order.html page, then the order form's reset button should be changed to .
In the following entry, we'll detail what happens when we click the order form's submit button.
Actually, reptile7's JavaScript blog is powered by Café La Llave. ;-)