reptile7's JavaScript blog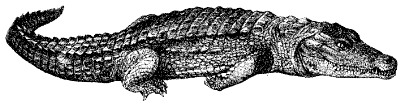
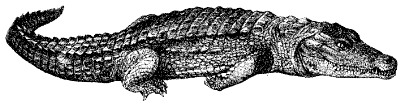
Saturday, July 28, 2007
JavaScript Cookies III
Blog Entry #83
First-time vs. return visitors
At the end of the previous post, we were discussing Script Tip #64's modified cookie script and the first if block of its putCookie( ) function:
cookie_name = "NameCookie2010"; var GuestName; var FirstTime;
function putCookie( ) {
if (document.cookie) {
index = document.cookie.indexOf(cookie_name);
FirstTime = "n"; }
In the "Place the Variable" section of Script Tip #64, Joe insinuates that if the document.cookie if condition returns true (
if a cookie exists) and if NameCookie2010 appears in the document.cookie return (
the index of the cookie was found,i.e., index is not equal to -1), then the user is a return visitor. True or false?
When I visit http://www.htmlgoodies.com/, the cookie below is placed on my hard disk:
RMID=04e708c346a3d1a0; domain=.htmlgoodies.com; path=/; expires=Friday, 31-Dec-2010 23:59:00 GMT
Because of its general domain=.htmlgoodies.com and path=/ settings, this cookie is associated with every page of the HTML Goodies site. Let's suppose that I surf to Script Tip #64 and test the Script Tips #60-64 Script's effect for the very first time via the "Try out the NEW Script" link; although this is my first visit to the Script Tip #64 Script demo page, document.cookie is not empty and converts to true vis-à-vis the if block above.
Pushing the envelope a bit, let's now suppose that http://www.htmlgoodies.com/ also writes to my hard disk the following cookie for keeping track of visitors to its "legacy" pages:
NameCookie2010=oldschool; domain=.htmlgoodies.com; path=/legacy; expires=Friday, 31-Dec-2010 23:59:00 GMT
For a first visit to the Script Tip #64 Script demo page/Script Tips #60-64 Script:
(a) document.cookie returns
NameCookie2010=oldschool; RMID=04e708c346a3d1a0*
and converts to true at the if (document.cookie) command line;
(b) document.cookie.indexOf(cookie_name) returns 0, which is assigned to index.
(*On my iMac, both MSIE and Netscape list cookies with a more specific path value prior to cookies with a less specific path value (domain values being equal) in the document.cookie string.)
For both of the preceding scenarios, "n" is assigned to FirstTime; in the former case, I will be greeted with putCookie's first prompt( ) box and will subsequently see Hello, (prompt( ) output), welcome back!!! on the page, whereas in the latter case, there won't be any prompt( ) dialog and I will see Hello, oldschool, welcome back!!! on the page, even though I am a first-time visitor in each case.
Cookies with the same name attribute value but different path attribute values are accepted individually by the browser - such cookies do not "override" one another - check out Example 2 at the end of the JavaScript 1.3 Client-Side Reference's "Netscape Cookies" Appendix.
OK, I admit it's unlikely that another HTML Goodies page would set a NameCookie2010 cookie. But here is my larger point: if you want to distinguish first-time and return visitors to a Web page via the Script Tip #64 Script or a related cookie script, then be sure that your tracking cookie has a sufficiently unique name attribute value.
The expires attribute value
Regarding a cookie's expires attribute value, Netscape's cookie property documentation says:
[A] valid cookie expiration date isActually, there are at least three other ways in which the above expires value format can differ from the return of the toGMTString( ) method of the Date object. On my computer when using MSIE, the toGMTString( ) return format is:
expires=Wednesday, 09-Nov-99 23:12:40 GMT
The cookie date format is the same as the date returned by toGMTString, with the following exceptions:
• Dashes are added between the day, month, and year.
• The year is a 2-digit value for cookies.
Tue, 9 Nov 1999 23:12:40 UTC
(November 9, 1999 was in fact a Tuesday.)
• The string begins with a three-letter abbreviation, and not the full name, of the day of the week.
• For the first nine days of a month, the string gives a 1-digit value for the day of the month.
• The string concludes with the UTC time zone designation.
Anyway, the question arises: if we attempt to set a cookie's expires value with the toGMTString( ) method -
var futureday = new Date("April 5, 2010");
var expiresday = futureday.toGMTString( );
document.cookie = "Cookie1Name=Cookie1Value; expires=" + expiresday;
- would that work, or would the browser throw an error? It'll work, say the folks at WebReference.com.
Netscape avers that
toGMTString( ) is no longer used and has been replaced by the toUTCString( ) method.Consistent with WebReference.com's earlier work, I find that the toUTCString( ) method can correspondingly be used to set a cookie expiration date with either MSIE or Netscape.
Extracting the value value, take 2
The Microsoft Developer Network's cookie property page provides an alternate, for-loop-based method for extracting the value attribute value of a cookie with a given name attribute value. MSDN's code is easily transplanted into the Script Tips #60-64 Script's getName( ) function:
function getName( ) {
// a two-character semicolon-space string separates each cookie in document.cookie
var aCookie = document.cookie.split("; ");
for (var i = 0; i < aCookie.length; i++) {
// a name/value pair is separated by an equal sign
var aCrumb = aCookie[i].split("=");
if (cookie_name == aCrumb[0])
return aCrumb[1]; } }
The function above uses the split( ) method of the String object to split
(a) document.cookie into its constituent cookies, and
(b) each document.cookie cookie into its name value and value value.
When the loop finds a match between cookie_name and aCrumb[0], then cookie_name's value value, which is held by aCrumb[1], is returned.
Alternate functions for the Script Tips #60-64 Script
Speaking of the getName( ) function, you've probably noticed that all of the getName( ) assignment statements also appear in the putCookie( ) function. I can see why the script's author set aside a separate function for determining the cookie_name value value, but given its redundant code, I myself would scrap getName( ) and remodularize the script according to the first-time-vs-return-visitor motif of the Script Tip #64 Script, as follows:
(1) First, let's retool the FirstTime conditional statements a bit and put them, along with the expires value, in the 'global' part of the first script element:
<script type="text/javascript">
var cookie_name = "myTotallyUniqueCookieName", FirstTime, GuestName;
if (document.cookie.indexOf(cookie_name) == -1) FirstTime = "y";
else FirstTime = "n";
var futureday = new Date("April 5, 2010");
var expiresday = futureday.toUTCString( );
(2) Next, let's replace the putCookie( ) function with newVisitor( ) and returnVisitor( ) functions:
function newVisitor( ) {
GuestName = window.prompt("Hello! What's your name?", "Nobody");
if (GuestName == null || GuestName == "") GuestName = "Nobody";
document.cookie = cookie_name + "=" + GuestName + "; expires=" + expiresday;
return GuestName; }
var index, namestart, nameend;
function returnVisitor( ) {
index = document.cookie.indexOf(cookie_name);
namestart = document.cookie.indexOf("=", index) + 1;
nameend = document.cookie.indexOf(";", index);
if (nameend == -1) nameend = document.cookie.length;
GuestName = document.cookie.substring(namestart, nameend);
if (GuestName == "Nobody") {
GuestName = window.prompt("Hello again!!!" + "\n"
+ "Last time you didn't tell me your name. Maybe you want to do it now?", "Nobody");
if (GuestName != "Nobody" && GuestName != null && GuestName != "")
document.cookie = cookie_name + "=" + GuestName + "; expires=" + expiresday;
else GuestName = "Nobody"; }
return GuestName; }
</script>
(3) Finally, the second script element can be recoded as:
<script type="text/javascript">
if (FirstTime == "y") document.write("Hello, " + newVisitor( ) + ", nice to meet you!!!");
else document.write("Hello, " + returnVisitor( ) + ", welcome back!!!");
</script>
Other HTML Goodies cookie tutorials
The Beyond HTML : JavaScript section of HTML Goodies features two cookie tutorials.
(1) "So, You Want To Set A Cookie, Huh?" offers a set-and-retrieve cookie script not that different from the Script Tips #60-63 Script; its demo may or may not work for you, as the URL paths to the demo page and to the tutorial main page are different.
(2) "So You Want A Cookie Counter, Huh?" offers a script (the tutorial's "Cookie Count Script" link is broken) that uses a cookie to count the number of times that a user has visited a Web page. In brief:
(a) The script sets a Counter_Cookie=1 cookie upon a first visit.
(b) Upon a second visit, the Counter_Cookie value value, 1, is extracted, converted from string to number via the eval( ) function, and incremented, and then a new Counter_Cookie=2 cookie is written, and so on for subsequent visits.
And that'll do it for our discussion of the Script Tips #60-64 cookie script(s) - good to the last crumb, eh? In the next entry, we'll wrap our tentacles around the Script Tips #65-68 Script, which displays colors corresponding to user-chosen color values.
reptile7
Wednesday, July 18, 2007
That's the Way the Cookie Script Crumbles
Blog Entry #82
For most of today's post we will deconstruct the cookie script of HTML Goodies' JavaScript Script Tips #60-63. The Script Tips #60-63 Script can actually be accessed - Land o' Goshen! - by following the "Here's the Code" links in all four script tips, and is reproduced in the div below:
<script language="javascript"> <!-- // This script was made by Giedrius gietam@eunet.lt cookie_name = "NameCookie2010"; var GuestName; function putCookie( ) { if (document.cookie) { index = document.cookie.indexOf(cookie_name); } else { index = -1; } if (index == -1) { GuestName = window.prompt("Hello! What's your name?","Nobody"); if (GuestName == null) GuestName = "Nobody"; document.cookie = cookie_name + "=" + GuestName + "; expires=Tuesday, 05-Apr-2010 05:00:00 GMT"; } else { namestart = (document.cookie.indexOf("=", index) + 1); nameend = document.cookie.indexOf(";", index); if (nameend == -1) { nameend = document.cookie.length; } GuestName = document.cookie.substring(namestart, nameend); if (GuestName == "Nobody") { GuestName = window.prompt("Hello again!!!" + "\n" + "Last time you didn't tell me your name. Maybe you want to do it now?","Nobody"); if ((GuestName != "Nobody") && (GuestName != null)) { document.cookie = cookie_name + "=" + GuestName + "; expires=Tuesday, 05-Apr-2010 05:00:00 GMT"; } if (GuestName == null) GuestName = "Nobody"; } } } function getName( ) { if (document.cookie) { index = document.cookie.indexOf(cookie_name); if (index != -1) { namestart = (document.cookie.indexOf("=", index) + 1); nameend = document.cookie.indexOf(";", index); if (nameend == -1) { nameend = document.cookie.length; } GuestName = document.cookie.substring(namestart, nameend); return GuestName; } } } putCookie( ); GuestName = getName( ); //STOP HIDING THE SCRIPT--> </script> <!-- What is below will appear on the page itself --> <script> document.write("Hello, " + GuestName + ", nice to meet you!!!"); </script>
Joe provides a demo page for the Script Tips #60-63 Script here.
Let's get rolling, shall we? Scalpel please, nurse...
<script language="javascript">
cookie_name = "NameCookie2010";
var GuestName;
The Script Tips #60-63 Script will set on the user's hard disk a cookie whose name attribute value is NameCookie2010 and whose value attribute value is held in the script by the variable GuestName. We begin by assigning the NameCookie2010 string to the variable cookie_name; subsequently, GuestName is declared but not initialized.
putCookie( );
The action moves to the next-to-the-last command line of the script's first script element: a call for the putCookie( ) function.
function putCookie( ) {
if (document.cookie) {
Next we have an if statement whose condition tests if there are any cookies associated with the current document; recall from the previous post that the document.cookie expression returns those cookies with validly matching domain and path attribute values and that such cookies do not need to have been set by the current document. (The if line has the form of a property-detection conditional but is obviously not meant to test the browser's support for the cookie property, which was implemented in the very first versions of JavaScript and JScript and is recognized by all but the most ancient browsers.)
If none of the browser's cookies is valid, then document.cookie returns an empty string, which is converted to false in a Boolean context; conversely, a document.cookie string of one or more matching cookies would convert to true here. And if the if condition returns true...
index = document.cookie.indexOf(cookie_name); }
This line checks if cookie_name (NameCookie2010) is present in the document.cookie return; if so, the character index of the starting N in the document.cookie string is assigned to the variable index.
For example, if document.cookie returns:
(a) NameCookie2010=Joe; Cookie2Name=Cookie2Value, then
index will return 0;
(b) Cookie1Name=Cookie1Value; NameCookie2010=Joe, then
index will return 26;
(c) Cookie1Name=Cookie1Value, then
index will return -1.
else { index = -1; }
If document.cookie is 'empty' and the preceding if condition returns false, then -1 is assigned to index.
if (index == -1) {
GuestName = window.prompt("Hello! What's your name?","Nobody");
if (GuestName == null) GuestName = "Nobody";
document.cookie = cookie_name + "=" + GuestName
+ "; expires=Tuesday, 05-Apr-2010 05:00:00 GMT"; }
Whether or not there are any cookies associated with the current document, an index value of -1 signals that the user is a first-time visitor*, to which this if block applies.
(*The converse is not true: as we'll explain later, if the user is a first-time visitor, then it doesn't necessarily follow that index is -1.)

The user types into the prompt( ) input field a name that, after the OK button is clicked, is outputted/assigned to GuestName.
• If the user doesn't enter a name into the prompt( ) field but just clicks the OK button, then the presupplied string Nobody will be assigned to GuestName.
• If the user clicks the Cancel button, then the primitive value null is assigned to GuestName; in this case, the following if (GuestName == null) GuestName = "Nobody" command line assigns Nobody to GuestName.
• Contra Script Tip #61, Nobody is not assigned to GuestName if the user clears the prompt( ) field and clicks the OK button; rather, an empty string is assigned to GuestName in this case.
Second, a NameCookie2010 cookie is written to the user's hard disk: cookie_name, "=", GuestName, and "; expires=Tuesday, 05-Apr-2010 05:00:00 GMT" are concatenated and the resulting string is assigned to document.cookie. This statement differs from a normal assignment statement in that the NameCookie2010 cookie is added to, and does not overwrite, any previously existing document.cookie cookies.
NameCookie2010's expires attribute value features a four-digit year but otherwise conforms exactly to the expires format given in Netscape's cookie property documentation.
else {
The following else block executes if the document.cookie string contains a NameCookie2010 cookie. Its first few statements extract NameCookie2010's value value; if this value is Nobody, then the user is given another chance to offer a Name and write a new NameCookie2010=Name cookie.
namestart = (document.cookie.indexOf("=", index) + 1); /* The outer parentheses are unnecessary. */
The right side of this statement returns the character index in the document.cookie string that is one past the index of the first = character following index, the character index of NameCookie2010's starting N.
For example, if document.cookie returns
NameCookie2010=Joe; Cookie2Name=Cookie2Value, then
index returns 0 and
document.cookie.indexOf("=", index)+1 will return 15.
The document.cookie.indexOf("=", index)+1 expression thus gives the position of the first character of NameCookie2010's value value (J in the example above) - this index is assigned to the variable namestart.
nameend = document.cookie.indexOf(";", index);
The right side of this statement returns the character index in the document.cookie string of the first semicolon following the index index. For example, if document.cookie returns
NameCookie2010=Joe; Cookie2Name=Cookie2Value, then
document.cookie.indexOf(";", index) will return 18.
The document.cookie.indexOf(";", index) expression thus gives the index position one past the last character of NameCookie2010's value value - this index is assigned to the variable nameend.
if (nameend == -1) { nameend = document.cookie.length; }
This conditional comes into play if the NameCookie2010 cookie is the last cookie in the document.cookie return and consequently NameCookie2010's value value is not followed by a semicolon. (Contra Script Tip #61, this if statement has no connection to NameCookie2010's expires attribute, which is not returned in the document.cookie string.)
If, for example, document.cookie returns
Cookie1Name=Cookie1Value; NameCookie2010=Joe, then
document.cookie.length will return 44, which will be assigned to nameend.
As for the preceding line, nameend represents the index position** immediately following the last character of NameCookie2010's value value (**in this case a characterless position beyond the end of the string).
GuestName = document.cookie.substring(namestart, nameend);
This line returns, and assigns to GuestName, NameCookie2010's value value (Joe if we were to stay with the above examples).
if (GuestName == "Nobody") {
GuestName = window.prompt("Hello again!!!" + "\n"
+ "Last time you didn't tell me your name. Maybe you want to do it now?","Nobody");
If GuestName is equal to Nobody (vide supra), then the prompt( ) box below pops up:

A \n newline may not induce a line break in most HTML settings, but as you can see, it definitely induces a line break on a prompt( ) box.
if ((GuestName != "Nobody") && (GuestName != null)) {
document.cookie = cookie_name + "=" + GuestName
+ "; expires=Tuesday, 05-Apr-2010 05:00:00 GMT"; }
If the user does not merely click the prompt( ) OK or Cancel button (as before, these responses assign respectively Nobody and null to GuestName) but changes the prompt( ) input field string in some way and clicks the OK button, then a new NameCookie2010=GuestName cookie is written/added to the document.cookie string.
FYI: in JavaScript, comparison operators have a higher precedence than logical operators, and thus the parentheses surrounding (GuestName != Nobody) and (GuestName != null) are unnecessary.
if (GuestName == null) GuestName = "Nobody"; } } }
The putCookie( ) function ends with an if statement that assigns Nobody to GuestName if the user clicks the Cancel button on the "Hello again!!!" prompt( ) box; as Joe points out in Script Tip #62, this ensures that the user will be reprompted for a name upon a return visit.
GuestName = getName( );
We move now to the last command line of the script's first script element: a call for the getName( ) function, whose output will be assigned to GuestName.
function getName( ) {
if (document.cookie) {
index = document.cookie.indexOf(cookie_name);
if (index != -1) {
• The if (document.cookie) { line and its concluding } right brace can be removed, because the document.cookie condition necessarily returns true for both first-time and return visitors.
• As in the putCookie( ) function, the index = document.cookie.indexOf(cookie_name) line locates the NameCookie2010 cookie in the document.cookie string and assigns the character index of the starting N to index.
• The if (index != -1) { line and its concluding } right brace can be removed, because the index != -1 condition necessarily returns true for both first-time and return visitors.
namestart = (document.cookie.indexOf("=", index) + 1);
nameend = document.cookie.indexOf(";", index);
if (nameend == -1) { nameend = document.cookie.length; }
GuestName = document.cookie.substring(namestart, nameend);
As in the putCookie( ) function, these statements extract and assign to GuestName the NameCookie2010 value value.
return GuestName; } } }
NameCookie2010's value value is returned to the GuestName = getName( ) command line and assigned once again to GuestName.
We're ready to greet the user by name via the script's second script element:
<script>
document.write("Hello, " + GuestName + ", nice to meet you!!!");
</script>
Perhaps you are wondering, "Why don't we put the getName( ) function call in the document.write( ) command?" - well, that would be more efficient, wouldn't it?
document.write("Hello, " + getName( ) + ", nice to meet you!!!");
The Script Tip #64 Script
The Script Tip #64 Script attempts to distinguish first-time and return visitors via a FirstTime variable and makes the following minor changes to the Script Tips #60-63 Script:
(1) A 'global' var FirstTime declaration is placed just before the function putCookie( ) declaration.
(2) A FirstTime = "n" statement for flagging a return visitor is added to putCookie( )'s first if statement.
(3) A FirstTime = "y" statement for flagging a first-time visitor is added to putCookie( )'s first else statement.
(4) The second script element is recast as:
<script language="javascript">
if (FirstTime == "y") { document.write("Hello, " + GuestName + ", nice to meet you!!!"); }
else { document.write("Hello, " + GuestName + ", welcome back!!!"); }
</script>
In theory, a first-time visitor is greeted with Hello, (prompt( ) output), nice to meet you!!, whereas a return visitor is greeted with Hello, (prompt( ) output), welcome back!!!, and in practice - check out the Script Tip #64 Script demo page here - this is what the user is likely to see. We noted earlier that a -1 index value definitely identifies a first-time visitor. However, the if (document.cookie) and index = document.cookie.indexOf(cookie_name) command lines cannot be used to unambiguously identify a return visitor, and I'll explain why in the next entry.
reptile7
Sunday, July 08, 2007
Adventures in Cookie Transmission
Blog Entry #81
If you're a typical Web surfer, then you probably have hundreds of cookies on your hard disk right now:

You are, no doubt, familiar with the common applications of cookies: for example, cookies are used by some Web sites to keep track of user IDs and passwords, and they play a starring role in e-business shopping carts; also and more controversially, some advertisers attempt to use cookies to build an 'online marketing profile' of you as you surf.
But perhaps you are a bit hazy as to what exactly cookies are and how they are placed on your computer. Regarding the former issue, HTML Goodies' "So, You Want A Cookie, Huh?" article says:
Cookies are a small computer-generated text file (no larger than 4K) that you receive when you stop into certain sites.There's some truth to this definition, but cookies are in fact strings comprising one or more attribute=value pairs delimited with semicolons, e.g.:
zGT=75S001; expires=Mon, 01-Sep-2008 23:52:00 GMT; path=/; domain=.nytimes.com
On my iMac, individual cookies are not housed in different files; Netscape loads its cookies into a cookies.txt file, whereas MSIE seems to internally store its cookies (or at least I can't find a separate MSIE 'cookie file' on my hard disk).
Regarding the setting of cookies on user machines, this is almost always done on the server side via a CGI script. Wikipedia nicely summarizes a normal cookie transmission process here. However, on the client side we can with JavaScript set cookies with much (but not all) of the functionality of HTTP Cookies. Way back in Blog Entry #13, we briefly discussed the cookie property of the document object. In today's post, we lay some groundwork for an analysis in the next post of a script that reads and writes the document cookie property and that spans HTML Goodies' JavaScript Script Tips #60, #61, #62, #63, and #64.
The document.cookie return
Before we tuck into the Script Tips #60-64 Script, it is necessary to clarify what is and is not returned when we read the document.cookie expression.
The syntax of a JavaScript cookie is given by the "Netscape Cookies" Appendix (C) of the JavaScript 1.3 Client-Side Reference:
name=value [;EXPIRES=dateValue] [;DOMAIN=domainName] [;PATH=pathName] [;SECURE]
A cookie necessarily has a name=value parameter and can optionally have expires, domain, path, and secure attributes. The document.cookie expression only returns the name=value parameter, even if all of the other attributes are specified; for example,
document.write(document.cookie);
outputs:
cat=meow; dog=bark; parakeet=chirp
Note that the name=value pairs are delimited with semicolons and that the last name=value pair is not followed by a semicolon.
Netscape's short definition of the cookie property -
String value representing all of the cookies associated with this document- suggests that the document.cookie expression only returns cookies that have been set by the current document, but I find that this is too narrow a reading of the word "associated".
When I visit http://www.earthlink.net/, two cookies are placed on my hard disk:
s_cc=true; domain=.earthlink.net; path=/
s_sq=%5B%5BB%5D%5D; domain=.earthlink.net; path=/
If I upload a cookietestA.html file to my http://home.earthlink.net/~reptile7jr/ EarthLink server space, then a document.write(document.cookie) command in the cookietestA.html document picks up the two http://www.earthlink.net/ cookies, evidently because these cookies "pass" domain matching and path matching due to their general .earthlink.net and / settings for the domain and path attributes, respectively.
Conversely, suppose in the cookietestA.html document we write the cookies
document.cookie = "chips=ahoy; domain=.earthlink.net; path=/";
document.cookie = "fig=newton; path=/";
document.cookie = "nutter=butter; domain=.earthlink.net";
and then go back to http://www.earthlink.net/ and type javascript:alert("Cookies: " + document.cookie) in the browser address bar and hit the return key - what do we see?
When using MSIE, the alert( ) box displays:
Cookies: s_cc=true; s_sq=%5B%5BB%5D%5D; chips=ahoy; nutter=butter
When using Netscape, the alert( ) box displays:
Cookies: s_cc=true; s_sq=%5B%5BB%5D%5D; chips=ahoy
Both alert( ) box displays include the chips=ahoy string because the original chips=ahoy cookie was equipped with domain=.earthlink.net and path=/ parameters.
The fig=newton cookie does not have a domain attribute, whose value in this case defaults to the home.earthlink.net hostname of the server that set it, which does not match the earthlink.net domain name of the current www.earthlink.net host; consequently, the fig=newton cookie is "invalid" vis-à-vis http://www.earthlink.net/'s server and is not picked up by either browser.
The nutter=butter cookie lacks a path attribute, whose value in this case should default to /~reptile7jr, and this is what happens when using Netscape, which does not return nutter=butter in the document.cookie string because, in essence, the /~reptile7jr path is 'too specific' for http://www.earthlink.net/. In contrast, MSIE sets the nutter=butter path value to /; path matching now succeeds and nutter=butter is returned.
Finally, let's set in cookietestA.html one more cookie lacking both the domain and path attributes -
document.cookie = "pecan=sandies";
- and let's upload a cookietestB.html file to the http://home.earthlink.net/~reptile7jr/ directory. It should now be clear to you that, for both MSIE and Netscape, pecan=sandies will not be recognized at http://www.earthlink.net/ but will appear in cookietestB.html's document.cookie return.
Got all that? Good. Moving on...
Script Tips #60-63 cover a main script that
(a) tests if a cookie with a particular name (or any other cookie) is "associated" with the current document; if not, the script
(b) writes a cookie with that name and a value taken from a preceding prompt( ) statement;
then, the cookie's value
(c) is extracted via String object methods (remember, cookies are strings) and
(d) inserted into a greeting on the page for the user.
Script Tip #64 presents a slightly modified script that, depending on the outcome of the part-(a) test, posts different greetings for first-time and return visits.
We'll go through the nonredundant parts of these scripts in the following entry.
reptile7
Actually, reptile7's JavaScript blog is powered by Café La Llave. ;-)