reptile7's JavaScript blog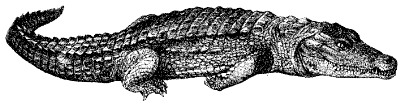
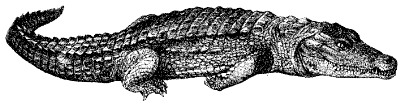
Saturday, November 19, 2005
More on Objects and JavaScript
Blog Entry #22
Ah, back in the Big Easy...after my whinging review of the DevGuru redesign in Blog Entry #20, let's get back on track, shall we? If you've been following along, you've caught on that there is in JavaScript a hierarchy of objects that mirrors the structure of an HTML document. If a document body element A contains an element B, for example:
<form name="A"><input name="B"></form>
then A and B are represented generally in JavaScript by objects that have a 'parent-child relationship': the text object B is the 'child' and is a JavaScript property of the form object A, which in turn is the 'parent' of B. We have also seen that this parent-child concept can apply to JavaScript objects that do not have direct HTML equivalents (window and history, window and location, etc.). Joe Burns devotes HTML Goodies' JavaScript Primers #8 to the hierarchy of JavaScript objects, which he illustrates, in simplified fashion, with the graphic below:

Joe breathlessly avers that the hierarchy of JavaScript objects is "THE secret to understanding JavaScript...once you understand this, you've conquered JavaScript!" I would respectfully disagree, and after doing some reading on the subject, I've got an even bigger bombshell to drop: the objects that are discussed in Primer #8 are no longer part of JavaScript.
They were at one time. Joe doesn't provide any references in his work, but I would bet that his graphic above is derived from Netscape's "Navigator Object Hierarchy", pictured below:

The Netscape graphic above, in turn, appears in Chapter 11 ("Using Navigator Objects") of the JavaScript 1.3 Client-Side Guide, which is in the "Obsolete Manuals" section of Netscape's DevEdge Manuals Web page. These "navigator objects", the "host objects" that we briefly discussed at the beginning of Blog Entry #5, are fleshed out in Chapter 1 ("Objects, Methods, and Properties") of Netscape's JavaScript 1.3 Client-Side Reference, but they do not appear in Netscape's JavaScript 1.4 Core Reference or JavaScript 1.5 Core Reference, nor do they appear in the specification for ECMAScript, the 'standardized' version of JavaScript. (JavaScript 1.5 is the latest official version of the language; JavaScript 2.0 is currently under development.)
So then: where does that leave these objects, now that Netscape has 'disowned' them? Glad you asked! They now belong to the "document object model (DOM)" - "model" as in "a schematic description of a system, theory, or phenomenon that accounts for its known or inferred properties and may be used for further study of its characteristics", to quote www.dictionary.com. As noted by Wikipedia, the DOM is an "application programming interface", allowing JavaScript and other programming languages (e.g., VBScript and Java) to access and make use of objects corresponding to HTML and XML elements.
(As Frank Zappa once said in another context:
"A novelist invents a character. If the character is a good one, he takes on a life of his own. Why should he get to go to only one party? He could pop up anytime in a future novel."
- The Real Frank Zappa Book, p 139)
The best discussion of the DOM that I've seen on the Web is by the Web Developer's Virtual Library (WDVL), whose "The Document Object Model Dissected" is very readable and worth reading, even if it is a bit dated and its links are mostly broken. At HTML Goodies, the DOM is briefly mentioned in Script Tip #23, but is otherwise not discussed. Weekend Silicon Warriors without a 'global programming perspective' are likely to find the official W3C DOM Level 1 Specification "a long, hard slog".
The prescriptive hierarchy of objects, and the command statements derived therefrom, originated with Netscape but are now also a part of the DOM. These statements - as we have seen in previous posts, as discussed at the start of this entry, and as noted in Primer #8 - take the form:
objectA.objectB.objectC.property_or_method
in which objectC is smaller than and contained by objectB, and is a property of objectB; and objectB is smaller than and contained by objectA, and is a property of objectA. Been there, done that.
We have already covered much of Primer #8's material:
• We discussed the window object, including its various 'aliases', in Blog Entry #18. In the "Deconstructing the Hierarchy of Objects" section of Primer #8, Joe states that "Windows [sic] is just an Object", but as we have seen, there is indeed a window property of the window object.
• The syntax for Image object command statements was given in Blog Entry #15. We didn't write the Image src (not "SCR") property in that entry, but we'll do so in the future.
• We dealt with the Location object and location.href statements in Blog Entry #19. Joe insinuates that the document and Location objects are related because they are at the same "level" of the object hierarchy, but this is only indirectly true in that both of these objects pertain to and are properties of the same parent window object.
• As far back as Blog Entry #2, we noted that a document is an object; that the document object is a property of the window object is discussed in the "Parts of a Window" section of Blog Entry #18.
• We treated the dual property/object nature of forms in Blog Entry #16.
• The command statements and value properties of form control objects were thrashed out in some detail in Blog Entry #17.
The Value Property
In Primer #8, Joe describes the value property of a form control object "as a reading of what something is or is not at a specific time." This vague statement is fair enough as far as it goes, but Joe makes some other comments about form control values that need to be clarified:
(1) Although there is no "value" node in his object "tree" above*, Joe states, "Note that Value and SRC are just Properties!" Not quite. We'll see in future primers that the value of a field-type control (text, textarea, etc.) functions in effect as a JavaScript String object, whose length property can be useful in data validation. (As you would infer from the preceding Netscape link, the String object is not part of the DOM but is a bona fide, built-in JavaScript object.) For example, consider the text box below:
whose coding is:
<form name="myform">
Enter your zip code, please: <input name="mytext">
</form>
<!-- Note that for an input element, the default type is "text". -->
A document.myform.mytext.value.length command would return, and thus check the correctness of, the number of digits entered by the user.
(*An even-more-condensed "Hierarchy of Objects" with a value node does appear in the Primer #8 Assignment.)
(2) "A checkbox can have a value of on or off depending if it's been clicked." Actually, as we saw in Blog Entry #17, the on/off nature of a radio button or checkbox is determined by the Boolean checked property, which is unrelated to the value property of these controls.
(3) "A TEXT field can have a value of hidden if you don't want the user to see it." Joe is referring here to the Hidden object, which corresponds to an <input> element whose type, and not value, is "hidden".
Method Examples
Each of the hierarchy references that we have seen thus far has terminated with a property; none has ended with a method. Let's wrap up this entry with demonstrations of the now-also-part-of-the-DOM focus( ) and select( ) methods, which are methods of the text, textarea, and password controls and which, in Blog Entry #10 in the midst of our introduction to event handlers, I said I would "illustrate...when we discuss the object hierarchy statements of forms in Primer #8."
The code for this is:
<form name="myform1">
<input name="mytext1" value="This is text box #1.">
<input name="mytext2" value="This is text box #2.">
<p>
<input type="button" value="Click here to bring focus to text box #1." onclick="document.myform1.mytext1.focus( );"><br>
<input type="button" value="Click here to select the value of text box #2." onclick="document.myform1.mytext2.select( );">
</form>
(Perhaps we should have done this back in Blog Entry #17, but better now than never, eh?)
HTML Goodies' JavaScript Primers #9, which we'll address in the next entry, will introduce us to JavaScript functions, an extension of the method concept that will allow us to execute an entire package of commands via a single event. Finally, let me note before signing off that I've been checking my links to DevGuru Web pages in previous posts, and they are now all functional...ausgezeichnet...whoever it was at DevGuru who 'reactivated' the old-school pages, thank you.
reptile7
Actually, reptile7's JavaScript blog is powered by Café La Llave. ;-)