reptile7's JavaScript blog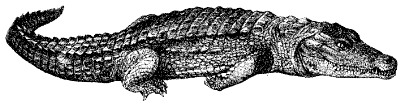
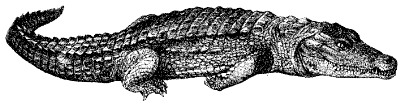
Sunday, March 27, 2005
Primer #1
Blog Entry #2
Hmmm...with my current system, I am evidently not going to be able to make use of Blogger's vaunted WYSIWYG post editor for writing my blog entries. According to the Blogger Browser Matrix, Blogger's Compose mode is supported by Netscape 7.2 for the Mac, which requires a more advanced operating system, a faster processor, and more physical RAM than my computer currently has. I do have Netscape 7.02 on my machine, but my attempts to bring 'focus' to the Compose textarea field using Netscape 7.02 are unsuccessful...it would thus appear, friends and neighbors, that I will have to hand-code my own blog-entry HTML. Horrors! But this is probably all for the better; otherwise, my HTML knowledge, such as it is, will get rusty. As an aside, in HTMLGoodies' introductory HTML primer, Joe Burns notes that he himself codes using the NotePad text editor, and not an HTML editor, most of the time.
We move on to our topic du jour, HTMLGoodies’ JavaScript Primers #1. Let's start by 'surveying the landscape' a bit. JavaScript is a computer language that, like human languages (English, French, etc.), has a syntax and various parts of speech. The three fundamental parts of speech of JavaScript are:
1) objects, which are analogous to nouns;
2) methods, which are analogous to verbs; and
3) properties, which are analogous to adjectives.
Speaking of JavaScript objects, Joe notes in this first primer that JavaScript coding is an example of object-oriented programming (OOP). A full discussion of OOP is definitely beyond the scope of this blog; I refer you to either Wikipedia's involved entry therefor or a somewhat more readable definition at Whatis.com. (Also, there used to be a technical Journal of Object-Oriented Programming, FWIW.) For our purposes, suffice it to say that in JavaScript we'll be using and manipulating "objects" on a regular basis.
Getting back to the primer, Joe introduces a first object, the "document" object, and a first method, the "write" method. The syntax for combining an object and method to form a JavaScript 'sentence', or command statement, is:
object.method( )
giving us in this case:
document.write("write this to my page")
in which the quoted code "write this to my page" - which can be text, HTML, or a combination thereof (as in the primer example) - in the write( ) parentheses is written to a Web page. Pretty simple.
In my first blog entry, I noted that there are some similarities between JavaScript and elementary algebra. In the example above, think of the "write this to my page" parameter of the write( ) method as an 'independent variable' that is acted on by the document.write( ) 'function', giving a 'dependent variable' in the form of the visual display of "write this to my page" on a Web page. At least that's how I look at it.
At the end of the primer, Joe provides definitions of the document object and the write( ) method on a "Click Here For What You've Learned" page. I'm not quite happy with his "document" definition, which reads, "This is an object name that refers specifically to the HTML document that contains the Javascript." More precisely, IMHO, "document" refers to the display, by a Web browser, of the document containing the JavaScript. Think about it: a document.write( ) statement in a JavaScript script in a "whatever.html" file on your computer or server doesn't post text/HTML to the whatever.html file, or to the code it contains - it posts to the display of whatever.html. This will make more sense when we get to some of the properties of the document object.
Speaking of properties, I'm a bit disappointed that there's no mention at all of JavaScript properties in this first primer. Just for illustrative purposes, Joe could have sneaked in a cameo by the bgColor property of the document object (analogous to the bgcolor attribute of the <body> tag in HTML), or he could have mentioned that the document object is itself a property of the "top-level" window object (not all JavaScript objects are created equal, as we'll see), but no matter - both the bgColor property and the window object are introduced in the 4th HTMLGoodies JavaScript primer.
Quick comments on other issues that crop up in the primer
The <script> tag
Joe informs us that "All JavaScripts start with [the] exact command":
<SCRIPT LANGUAGE="JavaScript">
Actually, with the advent of HTML 4.0, the language attribute of the <script> tag has been deprecated; the new standard is to use the type attribute, with a value of "text/javascript", instead, i.e.:
<script type="text/javascript">
(However, I confess that I myself find <script language="javascript"> easier to remember and will continue to use it until forced to change.)
Shape of the script, margins, and whitespace
According to Joe, "The major difference between [HTML and JavaScript] is that HTML is very forgiving in terms of its shape...the opposite is true in JavaScript." He warns against the careless use of whitespace and stresses that "you can not allow margins to get in the way". Well, yes and no.
I certainly agree that it is best to write JavaScript with a text editor (NotePad or, in my case, Apple's SimpleText) as opposed to some other type of file format (e.g., a word processor such as Microsoft Word) that has formatting/margin information operating behind the scenes.
As far as whitespace goes, I have found that simply adding extra spaces with the space bar, even lots of extra spaces, has no effect on the execution of a JavaScript script. (But then, I always write using SimpleText; this could be different with a word processor.) Line-breaks, generated by the return/enter key, are trickier; they're OK in some places but not in others, and it's not always intuitively obvious where you can put them without generating an error. Consider the primer example:
<SCRIPT LANGUAGE="javascript">
document.write
("<FONT COLOR='RED'>This Is Red Text</FONT>")
</SCRIPT>
Each line above is terminated by a line-break. Now, I can see line-breaks after LANGUAGE="javascript">, Text</FONT>"), and </SCRIPT>, but after write? I would've predicted truncation here to be problematic.
However, I did generate errors when I inserted line-breaks after various space characters in the script. For example, upon breaking the ("<FONT COLOR='RED'>This Is Red Text</FONT>") line in two by hitting Return just before the word Red:
("<FONT COLOR='RED'>This Is
Red Text</FONT>")
an "Unterminated string constant" "compilation error" popped up. We'll have more to say about errors in HTMLGoodies’ JavaScript Primers #2. Anyway, be careful with line-breaks.
One last point relating to this topic: a JavaScript script, like an HTML document, can definitely be written, if somewhat awkwardly, as one long, continuous line if its syntax is correct (notwithstanding Joe's implication that it can't).
"Is JavaScript Case Sensitive? Yes."
We'll see that JavaScript is generally, but not 100%, case-sensitive.
Quote formatting
In the primer example, the entire parameter of the write( ) method is enclosed in double quotes, whereas the value ('RED') of the color attribute of the inner <font> tag is enclosed in single quotes. Joe summarizes, "Remember: Inside of double quotes... use single quotes." But it could actually also be the other way around, i.e., double quotes inside of single quotes:
document.write('<FONT COLOR="RED">This Is Red Text</FONT>')
Either quote format works just fine - try it yourself. (And although it's a bit sloppy, the script will also work without any quotes at all around the "RED" value, not that I'm encouraging HTML sloppiness on anyone's part, of course.) Joe correctly notes that using double quotes inside of double quotes will cause the script to "think it has met the end of the line and...that will throw an error" (as will using single quotes inside of single quotes).
The end-of-primer assignment and its answer
Joe asks the reader to write "two lines of text, one red and one blue. But you must do this by writing more Javascript commands, not by simply adding more HTML to the instance [write( ) parameter]." I briefly note that the script in question can indeed be written with a single document.write( ) command statement and, as noted above, as one long line:
<script language="javascript">document.write("<font color='red'>This is red text</font><br><font color='blue'>This is blue text</font>")</script>
(Per our earlier discussion, there is in fact no line-break in the above code.)
That'll do it for our discussion of Primer #1. We'll tuck into the wonderful world of JavaScript error messages in Primer #2 next time.
reptile7
Friday, March 18, 2005
Intro
March 2005
"A writer is someone for whom writing is more difficult than it is for other people."
- Thomas Mann
Hello, everyone, and welcome to my blog. These are my first, tentative steps into the ‘blogosphere’ - wish me luck.
In general terms, I plan for this blog to be about programming for the Web, but I actually have a much more specific focus in mind: my blog entries, at least for the short term, will comprise a detailed, running commentary on my study of the JavaScript scripts at HTMLGoodies.
I have written to Joe Burns, the founder of HTMLGoodies, and informed him that I would launch such a blog. As of this writing, he hasn’t replied to me, so I can’t claim Joe’s ‘blessing’ for this project, but on the other hand, he hasn’t written back threatening to sue me, so onward we go.
I have no formal training in the use or programming of computers, although I’ve been using computers since the late 1980s when I was a graduate student. Today, like many people, I spend a lot of time on the Web, and it occurred to me, "Y’know, maybe I should learn something, at least a little bit, about how programming for the Web is done." Accordingly, I began a course of self-instruction in this regard with an article in the Fall 2001 issue of EarthLink’s now-defunct bLink magazine, "The ABCs of HTML" (p 32). (I’ll have more to say about EarthLink, my ISP, and instructional material relating to the Web at a later time.) This short, two-page introductory article on HTML concluded with a URL (www.earthlink.net/blink/aug99/workshop.html) for "our [EarthLink’s] HTML tag reference" that annoyingly led to a "Page Not Found" page.
I emailed EarthLink’s customer support, asking "Does EarthLink still have a reference page on HTML tags and/or attributes on its Web site, and if so, can you please send me its URL? Thank you." The response: "With regards to your inquiry about the given site, we are very sorry to report that we have no more reference page [sic] on HTML tags. To help you, you may use the search tool on http://www.google.com to get URLs about HTML tags." Pretty lame for an ISP of EarthLink’s stature, wouldn’t you say? But go to Google I did in my quest to learn more.
I soon found my way to HTMLGoodies. There are, to be sure, lots of sites on the Web that offer instructional material on HTML, but what sets HTMLGoodies apart, it seems to me, is that HTMLGoodies ‘assumes the least’ about a user’s beginning level of coding knowledge; in other words, it’s the most elementary of all the Web programming instruction sites that I’ve come across. Because I myself was starting from absolute scratch, I thus decided to have a go at HTMLGoodies ‘program’ and see what I could get out of it.
Since then, I have worked through the introductory primers and many of the accompanying tutorials on HTML and JavaScript at the HTMLGoodies site. Looking back, I have to confess that I did not find HTML, as a subject of study, to be particularly interesting; as its name implies, you take some text and then ‘mark it up’ so that it can be read by a Web browser - it’s sort of like proofreading, actually. But JavaScript was where this all took off for me. I immediately latched onto the algebraic nature of JavaScript - I liked algebra in "middle school" ("junior high school" in my case), so it stands to reason that I would like JavaScript. If you are comfortable with the concepts of variables and functions, both of which crop up in JavaScript, then you, too, may find JavaScript to be your cup of tea.
The JavaScript material at HTMLGoodies can be subdivided into three parts:
1) It begins with a series of 30 introductory Primers.
2) The Primers are supplemented with a series of "Script Tips".
3) Rounding things out is a collection of relatively more advanced tutorials.
As noted above, my blog will cover this material (or at least the Primers and Script Tips) in some depth.
Joe Burns and his JavaScript co-author Andree Growney have also written a book, JavaScript Goodies, that "is based on the most popular JavaScript tutorials at HTMLGoodies.com", according to Amazon.com - I myself haven’t seen/read it. (Perversely, Joe’s link to this book in JavaScript Primers #30 is broken.) You can see for yourself that the reviews of JavaScript Goodies are fairly mixed. Some folks think that Joe and his JavaScript work are the greatest thing since sliced bread, whereas others, particularly the hardcore computer-techie types, don’t like them at all. I myself can see both points of view. Most reviewers, even the critical ones, agree, in accord with my earlier comments, that the HTMLGoodies JavaScript material is a good starting point if you’ve never done any programming before. On the other hand, Joe can be sloppy, and he maddeningly seems to not check his work a lot of the time. I can confirm that if you are an obsessive-compulsive, anal-retentive, detail-oriented sort of person (and I admit that I myself can be like this), then you are guaranteed to be annoyed by the JavaScript material at HTMLGoodies on at least a semi-regular basis.
Having said this, however, there is very much a limit as to how many stones I’m willing to throw at Joe. After all, Joe was the one who put all this stuff on the Web, where it can be accessed by people like me for free, in the first place. If I at times level criticism at Joe and HTMLGoodies, it is very much in the spirit of Sir Isaac Newton’s famous quote, "If I have seen further than others, it is by standing upon the shoulders of giants."
For anyone who might read or follow this blog, let me repeat for the record that I am NOT a professional programmer, nor any sort of ‘expert’; I am a hobbyist. My own inexperience is to blame if I say something that is confusing or wrong. Comments that can bring me up-to-speed on a particular point are always welcome, and if I can make heads or tails of what you’ve said, then I may discuss it in the blog and hopefully make it a "teaching moment" for us all.
Let’s get rolling, shall we? Before getting started, I want to give credit where credit is due and mention a couple of external Web sites that have helped me get through the HTMLGoodies JavaScript scripts:
1) I have found the JavaScript resource at DevGuru to be essential, even if I find some of its examples to be confusing.
2) The folks at JavaScript Kit also run a highly useful site.
Hyperlinks to other resources will be provided where relevant.
Some final notes on my ‘Web interface’:
- my computer is a vintage iMac 350 whose currently installed operating system is Mac OS 9.1; (old school, I know)
- as noted above, EarthLink connects me to the Internet;
- my preferred Web browser is Internet Explorer* (version on my machine: 5.1.6), although blogger.com is going to get me back into using Netscape.
(*I’m sure this disqualifies me from being a full-fledged member of the Cult of Mac; I also use Outlook Express as my email client, BTW.)
Speaking of platforms and browsers, I recognize that JavaScript can execute differently (a) on different browsers and also (b) on different versions of the same browser, so it wouldn’t surprise me that it will at times execute differently on different platforms. I consequently can’t promise you that what works on my computer will work on your computer if you are a non-Mac user, as you are statistically likely to be. As they say at LowEndMac, "Advice presented in good faith, but what works for one may not work for all."
OK, that’s it. In our next episode, we’ll take a brief look at HTMLGoodies’ JavaScript Primers #1. Do join us, won’t you?
reptile7
Actually, reptile7's JavaScript blog is powered by Café La Llave. ;-)