reptile7's JavaScript blog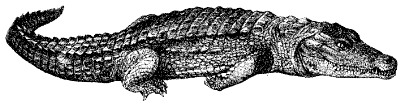
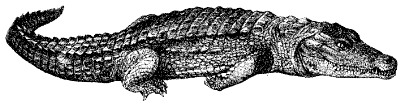
Thursday, May 26, 2005
Event Handlers, Act III
Blog Entry #10
We finish off our discussion of HTML Goodies JavaScript Primers #5 with some commentary on the following event handlers:
onSelect
onSubmit
onLoad
onUnload
onSelect
The onSelect event handler executes a JavaScript command statement in response to a select event, which refers not to the select form element but to the
highlighting of text characters in a text, textarea, or password form element with the mouse cursor. Consider, for example, the text box below; highlight with your mouse the "select me" text, and see what happens to it:
The coding for this is:
<form>
<input type="text" value="select me" onselect="style.fontVariant='small-caps';">
</form>
It's not necessary to select the entire box value to trigger the execution of the onSelect command; in fact, selecting just a single character will do it.
As far as I am aware, the onSelect event handler cannot be used with 'normal' text on a Web page; sure enough, my attempts to execute onSelect attribute commands in <p>, <span>, and <div> tags were unsuccessful.
Relatedly, JavaScript has a select( ) method that can be used to select (i.e., highlight) the fields of text, textarea, and password elements and, like the focus( ) method, thus prompt the user for input to these elements. We can illustrate these methods when we discuss the object hierarchy statements of forms in Primer #8.
onSubmit
A submit event occurs when a form is submitted by clicking a submit button form element. When using this event to execute JavaScript code via the onSubmit event handler, the onSubmit attribute should be put in the parent <form> tag, NOT in the <input type="submit"> tag, as incorrectly shown in the primer. We can demonstrate onSubmit with the form below; answer the question, and then click the submit button (if a dialog box pops up when you submit, click "Cancel"):
We will learn how to open new windows with JavaScript in Primer #11.
In this section of the primer, Joe demonstrates the onSubmit event handler with a new command:
onSubmit="parent.location='/legacy/primers/jsp/thanksalot.html';"
Joe's classification of "parent" as a property and of "location" as an object, while correct to an extent, is confusing and incomplete. Let's fill in the gaps, shall we?
"Parent" is serving here as a synonym of sorts for the window object (in Primer #8, it is defined as a "built-in name" for a window), and it is indeed classified as a property of the window object, but as noted by JavaScript Kit, outside of a frame context parent "simply refers to [the] current window". In other words, the command above can (and should, IMO) be formulated as window.location='/legacy/primers/jsp/thanksalot.html'.
As for "location", there is in JavaScript a location object, and as noted at DevGuru's location object page, the command above does in fact create a new instance of the location object, but in the command itself, location functions syntactically as a property of the window object; what the command does is assign a new value, in this case the URL of a different Web page, to this property, causing the browser window to move from the current page to the different page - it's "setting up a link", as Joe puts it. The command thus follows the object.property format that we have seen previously, and does not have a 'reversed' format, as the primer implies.
One more point - related to onSubmit is the JavaScript submit( ) method, which "[p]erforms the same action as clicking a submit button". Seems redundant, doesn't it? But there it is in the language for someone who might figure out a use for it.
onLoad
The onLoad event handler pertains most often to the window object. When you navigate to a new Web page with your browser, a load event occurs as you consequently load that page and its associated files temporarily into your computer's memory. It might then seem that onLoad would be associated with the document object - after all, it's the document that "loads" - but this is not the case. Anyway, the loading of Web pages can be tied to the execution of JavaScript code by placing an appropriate onLoad attribute command in the document <body> tag, as noted in the primer.
HTML Goodies waits until Primer #9 to illustrate the onLoad event handler, but that doesn't mean we can't sneak in a demo here, does it now? Click here to see an onLoad command in action; close the resulting window to see an onUnload command in action.
(I was originally going to put onLoad and onUnload attribute commands in the <body> tag of my Blogger template in order to demonstrate these event handlers directly here on my blog, but I found that I am unable to execute independently onSubmit and onUnload commands on my computer, so I created a separate page for onLoad and onUnload. I refer you to the source of the demo page for the coding.)
The onLoad event handler can also be used with an image element so as to execute JavaScript code when the image has fully loaded. We'll be working with images later on in the primer series, so perhaps we can revisit onLoad in an image context then.
onUnload
onUnload is an event handler only for the window object. An unload event occurs upon 'closing' a Web page in one way or another, e.g., going to a new page in the same window, closing the page with the Close command under the File menu, or quitting the browser. It follows that an onUnload command executes when leaving a Web page, and the onUnload attribute - like window-related onFocus, onBlur, and onLoad attributes - is placed in the <body> tag. HTML Goodies waits until Primer #10 to illustrate the onUnload event handler, using it there with a window.alert( ) command, as does the demo above.
I'll wrap up this entry with a few remarks about...
The end-of-primer assignment and its answer
Joe asks the reader to create an 'interactive' form whose individual elements each make use of an event handler.
Let's first note that a form with a text box, two checkboxes, and a submit button has four elements, and not three (each checkbox counts as a separate element).
Secondly, if we want the chocolate vs. vanilla preference to be an either/or choice, then we should use radio buttons, and not checkboxes, for this part of the form.
Thirdly, note that for the submit button, Joe uses an onClick event handler, and not onSubmit, in the <input type="submit"> tag to pop up an alert box when submitting the form (we could use an onSubmit command but it would go in the <form> tag, as noted above).
In Blog Entry #11, we'll check over HTML Goodies JavaScript Primers #6, which revisits the concept of variables and also introduces the prompt( ) method of the window object - see you then.
reptile7
Thursday, May 19, 2005
Event Handlers II
Blog Entry #9
After the detour of the last episode, we return to the HTML Goodies JavaScript primers, specifically, to Primer #5 in continuance of our discussion of event handlers that we began two entries ago. With onMouseOver now under our belt, we will in today's entry briefly look at Primer #5's first four event handlers:
onClick
onFocus
onBlur
onChange
(Joe outlines the procedure for internal linking in his "So, You Want A Page Jump, Huh?" tutorial. In doing this at Blogger, however, it is necessary for the target <a name="codeword"> anchor to have a closing </a> tag.
May 2016 Update: On my Intel iMac, the ice cream onFocus demo and the link onBlur demo work with Google Chrome but not with Firefox, Opera, or Safari; they worked with IE 5.1.6 back in the day but I don't know if they do or don't work with modern versions of IE.)
onClick
onClick has 'pride of place' among the event handlers, as it is the most widely applicable. Any document body element that can be (left-)clicked (even I as an old-school Mac user am using a two-button mouse these days) can hold an onClick attribute command statement in its HTML tag; for example, onClick can be used in tags for marking up text (as we saw in the last entry), links (as in the primer), images, and form elements.
In the "The onClick Command" section of the primer, a side issue crops up that is easily sorted out. Joe warns against the use of apostrophes in the alert( ) method parameter, which are "seen by the browser as the end of the text...Error." Curiously, Joe does not provide a link to his tutorial on escape characters allowing single and double quotes to appear in alert box messages. By using the backslash operator (\, appearing just above the return/enter key), we can type out the following code for a "You're off!" alert message:
<span style="color:blue;text-decoration:underline" onclick="window.alert('You\'re off!');">Click Here</span>
Try it out: Click Here
onFocus
When you 'select' a form element (e.g., a text box, textarea box, radio button, checkbox, etc.), either by clicking it or by moving to it via a tab operation, you bring "focus" to that element, and this focusing event can trigger the execution of a JavaScript command via the onFocus event handler. For example, consider the ice cream-related question below, and see what happens when you select the various flavors:
The coding for this is:
<form>
Which flavor of ice cream do you prefer?<br>
<input type="radio" name="ice_cream" onfocus="document.getElementById('van').style.background='oldlace';">
<span id="van"> Vanilla</span><br>
<input type="radio" name="ice_cream" onfocus="document.getElementById('choc').style.background='sandybrown';">
<span id="choc"> Chocolate</span><br>
<input type="radio" name="ice_cream" onfocus="document.getElementById('straw').style.background='hotpink';">
<span id="straw"> Strawberry</span>
</form>
In selecting the choices above by clicking them, you may be wondering, "Can't we just use onClick here, in place of onFocus?" Indeed we could, actually - in most cases involving form elements, the onFocus and onClick event handlers can be used interchangeably. The not-so-important difference between onFocus and onClick in this case is that we could, if we wanted to, bring focus to the "Chocolate" and "Strawberry" radio buttons without clicking them, that is, we can initially click the "Vanilla" button and then access the "Chocolate" and "Strawberry" buttons by using the tab key, but who would do that, really?
The onFocus event handler should not be paired with a window.alert( ) command; as DevGuru explains on its onFocus page, "Be aware that assigning an alert box to an object's onFocus event handler with [sic] result in recurrent alerts [i.e., the alert box will pop up over and over again, indefinitely] as pressing the 'o.k.' button in the alert box will return focus to the calling element or object." (In trying it out, I found this to be true when using Netscape but not MSIE, FWIW.)
onBlur
With respect to user actions, "blur" is sort of the opposite of "focus" in that a blur event occurs when focus is removed from an element. Like onFocus, the onBlur event handler is largely applicable to form elements. The primer suggests that when onBlur is used with either a text box or a textarea box, a change in the box "value" (the text appearing in the box) is required to execute an onBlur command, although this is not true; merely clicking outside of the box with focus, changed value or not, will do it.
I'll demonstrate the onBlur event handler with a simplified version of DevGuru's example. Click inside of the text box below, and then outside of it:
The code for this is:
<form>
Enter your email address, please: <input type="text" size="40" onblur="window.alert('Please check that your email details are correct before submitting.');">
</form>
Less intuitively, focus and blur events can also apply to links. In illustration, click on the left text box below, and then press the tab key to access the central link, and then press the tab key again to access the right text box:
The code for this is:
<form>
<input type="text"> <a href="#" onfocus="document.getElementById('main').style.background='peachpuff';" onblur="document.getElementById('main').style.background='lemonchiffon';">This is a link.</a> <input type="text">
</form>
On my computer, the link's focus, like that of the text boxes, is indicated by a surrounding colored (MSIE, with the color being set by the Browser Color command under the View menu) or gray and tinily dotted (Netscape) rectangle. Note that a link with focus is not the same as an "active" link - a link that is 'in transit' from the "source" anchor to the "target" anchor - whose color can be set, at least for the time being, by the now-deprecated alink attribute of the document <body> tag.
Lastly and more practically, focus can be brought to and removed from the browser window (the windows of Internet pop-under ads are "blurred", for example). The focusing or blurring of windows can execute JavaScript code by the use of onFocus and onBlur command statements, respectively, in the <body> tag.
Related to the onFocus and onBlur event handlers are the focus( ) and blur( ) methods, which are methods of the window object and of all of the form element objects and can be used to bring and remove focus to and from these objects. The focus( ) method will crop up in a form field validation script in Primer #29, near the end of the primer series.
onChange
Whereas onFocus and onBlur are usually used with form elements, the onChange event handler, to the best of my knowledge, is used exclusively with form elements. Of the commonly encountered form elements, the text, textarea, and select elements are those that can hold an onChange attribute in their HTML tags. For a text or textarea box, an onChange attribute command will execute upon changing the contents of the box, whether initially empty or not, and then clicking outside the box, as demonstrated in the primer for a text box. For a select element ("pop-up box"), any change in the currently selected option will execute an onChange attribute command. Try it out with the example below:
You get the idea. ;-) The code for this is:
<form>
Which flavor of ice cream do you prefer?<br>
<select onchange="window.alert('It\'s time for an ice cream cone, wouldn\'t you say?');">
<option>Butter Pecan</option>
<option>Cherry Vanilla</option>
<option>Mint Chocolate Chip</option>
<option>Rocky Road</option>
</select>
</form>
onChange is also an event handler for the file upload form element, to which onClick, onFocus, and onBlur also all apply. File upload elements are used by some Webmail services to attach files to emails (e.g., Excite, with whom I have an email account, uses them). As an aside, DevGuru shows some requirements for sending files to others via <input type="file"> elements here.
And onChange will work with the password form element, too, or at least it does on my computer. Type a fictitious password into the box below, and then click outside of the box:
The code for this is:
<form>
Enter your password, please: <input type="password" onchange="window.alert('Are you sure that your password is typed correctly?');">
</form>
The <input type="password"> tag can similarly hold onClick, onFocus, and onBlur attribute commands.
One would think that onChange might be usable with radio button and checkbox form elements. I experimented with this a bit, and I was able to achieve a semblance of interactivity, but the overall results were not very satisfying, except for one specific case: I found that I could use onChange with checkboxes reliably when using Netscape. If you're a Netscape user, try out the following demo on your system and see if it works:
<form>
What kind of pet do you have at home? (<em>Check all that apply.</em>)<br>
<input type="checkbox" onchange="window.alert('Meow!');"> Cat<br>
<input type="checkbox"> Dog<br>
<input type="checkbox"> Bird<br>
<input type="checkbox"> Snake<br>
</form>
(If it doesn't work, well, c'est la vie.)
BTW, dynamic style changes do not constitute change events. An attempt to apply onChange to a span element with the following code was unsuccessful:
<span onmouseover="style.background='yellow';" onchange="window.alert('The background is now yellow');">The rain in Spain falls mainly on the plain.</span>
I'll continue with the other event handlers of Primer #5 - onSelect, onSubmit, onLoad, and onUnload - in my next post.
reptile7
Tuesday, May 10, 2005
JavaScript, CSS, and Background Coloring
Blog Entry #8
JavaScript and cascading style sheets (CSS) intersect in today's entry to produce some nifty background-coloring effects.
Roll your mouse over the colors of the color series below:
Red Orange Yellow Green Blue Indigo Violet
(Those of you who are 'scientifically inclined' will recognize that these colors in the form of light approximately make up the visible region of the electromagnetic spectrum, which ranges from lowest energy (longest wavelength) red light through the intermediate colors of the series to highest energy (shortest wavelength) violet light. Acronym fans, remember: ROY G BIV.)
To 'statically' set a background color for a document element, we would put the attribute:
style = "background-color: colorinfo"
("colorinfo" is the desired color as a word description or hex code)
or, more simply:
style = "background: colorinfo"
into the relevant HTML tag, e.g., <p>, <span>, <div>, etc. (At HTML Goodies, discussion of the <span> tag appears here, whereas the <div> tag crops up in the "So, You Want Positioning, Huh?" tutorial.)
For example, <span style = "background-color: lightseagreen">The Pacific Ocean</span> gives: The Pacific Ocean
To set background colors dynamically is not much more difficult, and can be done by making use of the JavaScript style object; to do this by, say, a mouseover, we can use the following command statement:
onmouseover = "this.style.backgroundColor = 'colorinfo';"
(the "this" keyword is a special operator that refers to the document element in whose HTML tag it resides; in "Example 2" on the JavaScript Kit style object page, "this" refers specifically to a division element)
or, more simply:
onmouseover = "style.background = 'colorinfo';"
In these statements, "style" is simultaneously serving as (a) an object, with a backgroundColor (background) property whose value is to be 'colorinfo', and (b) a property of the "this" element.
So, getting back to the demo at the top of the page, the code for dynamically changing the background (and 'foreground') color of "Red" is:
<span onmouseover="style.background='red'; style.color='white';">Red</span>
Not so tough, eh?
The getElementById( ) method
In extension of the above, we can access and dynamically style any document body element whose HTML tag holds an id="whatever" attribute via the getElementById( ) method, a method of the document object and one of the most powerful methods in JavaScript. In illustration, click on the question below:
What is the capital of Nicaragua?
(A) San Salvador
(B) Tegucigalpa
(C) Managua
(D) San José
The "What is the capital of Nicaragua?" question constitutes a span element, whose <span> tag holds an onClick event handler, which is discussed in Primer #5; the correct answer, "(C) Managua", also constitutes a span element, whose <span> tag holds an id="niccap" attribute. Connecting the two is the following onClick command statement whose syntax is again helpfully provided by JavaScript Kit:
onClick="document.getElementById('niccap').style.backgroundColor='yellow';"
(again, the "Color" of "backgroundColor" can be dropped)
I trust that you can write out the complete code for all of this.
Blogger background colors, revisited
With the getElementById( ) method now part of our JavaScript armamentarium, we are ready to horse around with background colors here at Blogger.com.
This blog makes use of the (old-school, no-longer-available) "Bluebird" Blogger template.
Click here to view the source code of my template. Click here to hide the code.
In the template, a post is contained in a <$BlogItemBody$> template tag that sits in a division element whose <div> tag holds a class="Post" attribute; in turn, the <div class="Post"> division is part of a larger division element whose <div> tag holds an id="main" attribute. To set a new background color - say, violet - for the <div id="main"> division, we can straightforwardly write:
<span onclick="document.getElementById('main').style.background='violet';">Click on this sentence to change the post background color.</span>
Try it below:
Click on this sentence to change the post background color.
Similarly, the "About Me" section of the blog that occupies the upper-right-hand corner constitutes a division whose <div> tag holds an id="menu" attribute. To set a new background color - say, gold - for the <div id="menu"> division, we could write:
<span onclick="document.getElementById('menu').style.background='gold';">Click on this sentence to change the background color of the "About Me" section.</span>
Try it below:
Click on this sentence to change the background color of the "About Me" section.
Let's get back now to the document.bgColor command, which I discussed but did not demonstrate in my last blog entry. The background color of the document is set by the body selector that kicks off the inline style block at the top of the template source; as you can see, the body's background-color property has the value "#C3CFE5", corresponding to a pale blue, which colors the stripes running up and down the sides of the template. In trying to change the color of the background side stripes, the problem here is that the document.bgColor command will not override the body selector information. What to do? The solution, it turns out, is quite simple:
(1) Remove "background-color:#C3CFE5" from the body selector; in its stead, insert a bgcolor="#C3CFE5" attribute in the <body> tag.
(2) NOW use a document.bgColor command where desired to change the document background color. To change the side stripes to red, for example, we could write:
<span onmouseover="document.bgColor='red';">Move your mouse over this sentence to change the side-stripe color.</span>
Try it below:
Move your mouse over this sentence to change the side-stripe color.
Alternatively, we could insert an id attribute - say, id="sidestripes" - in the <body> tag and then use an onmouseover="document.getElementById('sidestripes').style.background='red';" command statement in the <span> tag.
I'm still having problems with writing new messages to the window status bar here at Blogger - when I go to the Blogger site, there seems to be something from the Blogger server that my computer won't load, and a "Transferring data from www.blogger.com... [or buttons.blogger.com...]" status message preempts the execution of the window.status.command, at least when I'm using Netscape - but at least we're all up to speed on background coloring with JavaScript. Next time, we'll get into some more event handlers as we take on HTML Goodies' JavaScript Primers #5.
reptile7
Tuesday, May 03, 2005
Event Handlers I
Blog Entry #7
One of the most useful aspects of JavaScript is that the execution of JavaScript commands can be triggered by various user actions, e.g., loading a Web page, clicking a link, moving the mouse, etc., which are in JavaScript collectively termed "events". JavaScript links a user action with a resulting command via a part of speech called an event handler, to which we turn our attention in both HTMLGoodies' JavaScript Primers #4 and #5. Primer #4 focuses on a single event handler, the onMouseOver event handler, which, as its name implies, executes a command when the mouse cursor is moved over a document element; Primer #5 will introduce eight further event handlers for our delectation. Parenthetically, there is in JavaScript an event object, which is created automatically on the occurrence of an event and which is recognized by later versions of MSIE and Netscape; the event object doesn't crop up anywhere in the HTMLGoodies JavaScript primers but does appear late in the script tip series, in the "Cat Chases Your Mouse" script of Script Tip #91.
(May 2016 Update: Browser support for the status property of the window object has pretty much collapsed, so don't be too heartbroken if the Primer #4 script doesn't work for you.)
Onward, now, to the onMouseOver event handler and the Primer #4 script that utilizes it:
<A HREF="http://www.htmlgoodies.com"
onMouseOver="window.status='Go to the Goodies Home Page';
return true">Click Here</A>
(The above code does not need to be put on one line; it works fine just as it is.)
You may be thinking to yourself, "This isn't a script at all...this onMouseOver thing is really just an attribute in an HTML tag", and you would be right, more or less - that's pretty much how event handlers function. (Event handlers can be put in JavaScript scripts, but the coding to do this is more complicated than that shown above, and it's just not necessary most of the time.)
The command that is 'assigned' to the event handler, and which will execute in response to the mouseover event, is:
window.status='Go to the Goodies Home Page';
In this command, "window" is a host object representing the browser window; "status", in turn, refers not so much to the status bar at the bottom of the window as it does to the information (text, a URL, etc.) appearing in the status bar - the status information is classified as a property of the window object. The syntax here can be generalized as:
object.property = "value to be assigned to the property"
which is both similar to and different than the object.method( ) syntax that was introduced in Primer #1. Formally, then, our onMouseOver command will assign the 'Go to the Goodies Home Page' text string to the status property of the window object; in practice, 'Go to the Goodies Home Page' will be written to the status bar when the mouse is moved over the "Click Here" link.
Q & A
Q: Can the onMouseOver event handler be used with other HTML tags besides an <a> (anchor) tag?
A: Most certainly - pretty much any tag for an element in the body of a document can hold the onMouseOver attribute.
Mouseover examples with span, img, and button elements
(i) Move your mouse over the red words to change their color and background color.
(ii) Move your mouse over Mr. Alligator to make him grow.

(iii) Now, roll it over the button below for an important message. (We'll cover alert( ) boxes here.)
Q: Must the onMouseOver capitalization pattern be followed?
A: No. Capitalization is not important for event handlers; to the best of my knowledge, this is the one situation to which JavaScript's case-sensitivity does not apply. "onMouseOver", "onMouseover", and "onmouseover" are all acceptable (even oNmOuSeoVEr will work, I can confirm).
Q: What is this "return true" business, and is it necessary?
A: In JavaScript, the word "return" is classified as a statement; it is typically used inside of a function (a 'packet' of ≥1 statements - we'll get to JavaScript functions in Primer #9) to return a value to the point on the page that 'calls' (triggers) the function. Here, "return" is serving an analogous purpose by returning to the onMouseOver event handler the boolean value "true", which guarantees that, yes, the window.status='Go to the Goodies Home Page' command statement will well and truly execute regardless of anything that might preempt it; specifically in this case, "return true" causes the window.status command to override the 'default HTML behavior' of displaying the "http://www.htmlgoodies.com" URL in the status bar that would ordinarily occur when the mouse moves over the link.
Joe's attempted explanation of "return true" in the primer - "[i]f the words are present, then the script checks to see if a status bar is present" - is really rather bizarre; clearly, the presence or absence of the status bar merely determines whether or not you'll see the 'Go to the Goodies Home Page' text string, and not whether the window.status command executes in the first place. But in fairness to Joe, neither DevGuru nor JavaScript Kit is helpful on this topic. The best account I've seen on the Web on the use of "return true" in setting the status property of the window object via the onMouseOver event handler appears in <Code_Punk>'s JavaScript Lesson 13, to which my discussion above is indebted.
Second question: is "return true" necessary? Not really, IMHO. Using MSIE, I found that "return true" has no effect at all; I took it out and the window.status command still 'locked' the 'Go to the Goodies Home Page' text string in the status bar, i.e., subsequent mouseovers did not replace 'Go to the Goodies Home Page' with the link URL. Using Netscape, however, "return true" was indeed necessary to lock in the string; without "return true", I saw the mouseover behavior described in the primer - initially the link URL displays in the status bar, then the window.status command executes when the mouse is moved off the link, with subsequent mouseovers showing the link URL again. It's a bit counterintuitive in that you would expect the mouseover event to coincide timewise with the execution of the window.status command, but it's not as though the window.status command doesn't 'fire' at all. Moreover, Joe confesses that he prefers to not override the normal status bar display of the URL when moving the mouse over a link, and this is my preference as well.
It follows from the above that if the onMouseOver="window.status='whatever';" attribute/value is incorporated into another, nonanchor tag that has no default behavior to override, then "return true" will not be necessary to lock the new status bar message, although if you have more than one window.status command on a page, then each window.status command will override the other(s), with or without "return true".
Other primer issues
The bgColor property
In the "Other Properties, Other Uses" section of the primer, Joe re-presents the script with a new onMouseOver command:
onMouseOver="document.bgColor='pink';"
As you would guess, "bgColor" is a property of the document object, and is used to set the background color of a document (more precisely, it sets the background color of the display of a document by a Web browser). Like the bgcolor attribute of the HTML <body> tag (and color-related attributes of other HTML tags, e.g., the color attribute of the <font> tag), the value of the bgColor document property can be in the form of either a word description (as in the command above) or a six-digit hexadecimal code (i.e., instead of 'pink', we could type 'ffc0cb', pink's "hex code").
In setting the document's background color by the mouseover event, there is no 'competition' between the execution of the document.bgColor command and the default display of the "http://www.htmlgoodies.com" link URL in the status bar; consequently, "return true" can be left out of the onMouseOver attribute value.
bgColor is also a property of the JavaScript layer object. Layers are specifically supported by the Netscape 4.x browser series (Netscape 6+ doesn't recognize them) and are thus not much use to us, but they do crop up in the script tips, and we can talk about them then.
Finally, you may know that the use of cascading style sheets (CSS) allows background coloring to be applied not just to the entire page but to various elements of a document. CSS-based background coloring can be dynamically set with JavaScript; the coding to do this is a bit more advanced than that shown in the Primer #4 script, but at the risk of getting somewhat ahead of ourselves, I may well flesh this out in my next blog entry.
Two onMouseOver commands at once
In the "But I Want Both Effects" section of the primer, Joe sets up the simultaneous execution of two onMouseOver commands with the following code:
<A HREF="http://www.htmlgoodies.com"
onMouseOver="document.bgColor='pink',
onMouseOver=window.status='Go to the Goodies Home Page';
return true">Click Here</A>
If you were to suspect that the format above is unnecessarily complicated, well, you would be right once again. Here's what I've found:
(1) Only one onMouseOver is necessary; it's not necessary to nest a second onMouseOver inside of the outlying onMouseOver attribute.
(2) The document.bgColor and window.status commands can be delimited with either a comma or (contra the primer) a semicolon.
(3) If you're going to include a "return true" statement, then it must be delimited from the rest of the attribute value with a semicolon (delimiting it with a comma will negate both of the preceding commands, regardless of how they're delimited).
Our streamlined code is thus:
<a href="http://www.htmlgoodies.com" onmouseover="document.bgColor='pink'; window.status='Go to the Goodies Home Page'; return true">Click Here</a>
I don't know if there's any limit to the number of commands that can be strung together in this manner, but in the absence of any 'conflict' between them, then they should all execute with a single mouseover, at least in theory.
One more point - if you were to have two separate onMouseOver attributes in the anchor tag:
<a href="http://www.htmlgoodies.com" onmouseover="document.bgColor='pink';" onmouseover="window.status='Go to the Goodies Home Page';">Click Here</a>
only the first onMouseOver command will execute; with the code above, you'd change the document background color but not the status bar message.
The end-of-primer assignment and its answer
Despite my best efforts, I have thus far been unsuccessful in manipulating my Blogger template code so as to allow me to show the execution of window.status and document.bgColor commands directly here on my blog. However, the end-of-primer assignment introduces a new command that I AM able to demonstrate: the window.alert( ) command. For example, move your mouse cursor over the sentence below:
Let's go for coffee.
As noted on the HTMLGoodies JavaScript methods keyword reference page but not in the primer, alert( ) is a method of the window object; as shown by my demo, it generates a box with (a) a message, which is supplied by the alert( ) parameter, and also (b) an OK button. In the example above and also in the primer assignment, the alert box displays a text string; it can also be used to display the values of object properties and variables. Consider the following code:
<span onmouseover="window.alert(document.bgColor)">What is the page's background color?</span>
in this case, an alert box displays the value of the document bgColor property as a hex code (#ffffff, or white) upon rolling the mouse over the question below:
What is the page's background color?
The alert( ) method does not recognize HTML tags, however:
window.alert("<font color='red'>alert box message</font>")
will not give you an alert box with red text; rather, the HTML will appear on the box along with the message.
You'll note in the primer assignment that the alert( ) method does not need to reference the window object, i.e., the "window." part has been left out - this is 'allowed', if a bit sloppy, because the window object is at the top of JavaScript's 'host object hierarchy', which we'll get to in Primer #8, and thus its "existence is assumed". Relatedly, the window.status = "message" command statement can also simply be written as status = "message".
I think that's quite enough for Primer #4, don't you? Before we get stuck into Primer #5, I have some more things to say about JavaScript and background coloring, and I'll do that in the next entry.
reptile7
Actually, reptile7's JavaScript blog is powered by Café La Llave. ;-)