reptile7's JavaScript blog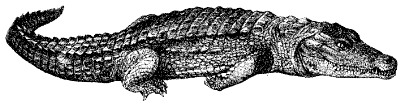
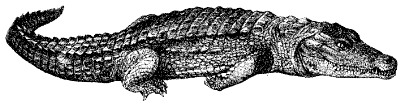
Wednesday, July 29, 2009
This Window Will Self-Destruct in Three Seconds
Blog Entry #152
In the two HTML Goodies "Opening New Windows with JavaScript" tutorials we've looked at so far, new windows are opened and closed via user events. The "Quick Window" tutorial uses a mouseover event to pop up a new window, whereas the "Remote Image" tutorial uses a click event to do so. The "Quick Window" script enables its window to be closed by a blur event, whereas the "Remote Image" script provides its window with a button that when clicked closes the window (if you close these windows in a 'normal' way, i.e., by clicking the close button in the window's title bar, by choosing the Close (Window/Tab) command under the File menu, or by typing Command-W or Control-W, these actions would still constitute user events, of course).
Today we move on to the "Hello Goodbye" tutorial, whose script pops up a small window with a greeting and two(-to-three) other lines of text

and then closes that window three seconds later without any user input at all. The "Hello Goodbye" script is reproduced in the div below:
<script type="text/javascript">
config = 'toolbar=no,location=no,directories=no,status=no,menubar=no,width=200,height=150';
config += 'scrollbars=no,resizable=no';
pop = window.open("", "pop", config);
pop.document.write('<script type="text/javascript">');
pop.document.write('setTimeout(');
pop.document.write('"self.close( )');
pop.document.write(';",3000)');
pop.document.write('</');
pop.document.write('script>');
pop.document.write('<body bgcolor="ltgreen">');
pop.document.write('<center><b><h2>Welcome</h2></b></center>');
pop.document.write('<center><b><h3>Please Wait... While This Page Loads</h3></b></center>');
pop.document.write('<center><b><h6>This Window Will Close Itself...</h6></b></center>');
pop.document.write('</body>');
</script>
As for the "Quick Window" and "Remote Image" scripts, Joe provides no discussion for the "Hello Goodbye" script, whose analysis is left to us and is given below.
Deconstruction
Opening the window
Unlike the "Quick Window" and "Remote Image" windows, the "Hello Goodbye" window is opened by a 'global' (outside of a function) window.open( ) command:
pop = window.open("", "pop", config);
The window's object reference will be pop and its name value will also be pop - to use "pop" as an identifier twice in this way strikes me as bad form, but it doesn't cause the script any problems.
Prior to the window.open( ) command are two statements that preassemble the command's strWindowFeatures parameter value, which is variabilized as config (hmmm...maybe this is where Joe got the config= that appears in the Primer #11 script):
config = 'toolbar=no,location=no,directories=no,status=no,menubar=no,width=200,height=150';
config += 'scrollbars=no,resizable=no';
The first of these lines sets the width and height of the window's viewport to 200 pixels and 150 pixels, respectively. The config lines also set seven other new window features to no, which is unnecessary, because
[i]f you define the strWindowFeatures parameter, then the features that are not listed or requested in the string will be disabled or removed (except titlebar and close*, which are by default [set to] yes),quoting Mozilla. Consequently, the window-opening code can easily be written as a single line:
var pop = window.open("", "pop2", "width=200,height=150");
I can confirm that the preceding command's effect is equivalent to that of the corresponding "Hello Goodbye" code for all of the OS X browsers on my computer; however, this doesn't mean that all of the non-size window features are in fact turned off, as we'll see later.
*The close feature - which relates to the browser window's close button and the File menu's Close command, and which is detailed in the "Features requiring privileges" subsection of Mozilla's window.open( ) page** - is not supported by Internet Explorer.
**Unfortunately, Mozilla does not code individual
<a name="featureName">featureName</a>
anchors for the various window.open( ) features (as Netscape did in the JavaScript 1.3 Client-Side Reference - check the source here), so follow the above quoting Mozilla link and then scroll down or use your browser's Find facility to get to the close feature.Closing the window
The remainder of the "Hello Goodbye" script comprises a set of eleven pop.document.write( ) commands that writes the document contained by the pop window. The first six of these commands build a script element itself holding a single command: a setTimeout( ) command that closes the pop window after a 3000-millisecond delay.
pop.document.write('<script type="text/javascript">');
pop.document.write('setTimeout(');
pop.document.write('"self.close( )');
pop.document.write(';",3000)');
pop.document.write('</');
pop.document.write('script>');
The setTimeout( ) script element is all we have for the pop document head - a title element, a required child of a document's head element, is not present.
I can see why the script element end-tag is split into </ and script> parts: as explained in the "Differences in JavaScript-generating HTML" subsection of Mozilla's "Migrate apps from Internet Explorer to Mozilla" article, this is another way of escaping the </ character sequence in a document.write( ) argument and thus preventing the browser from prematurely terminating the enclosing script (in this case, the "Hello Goodbye" script as a whole). However, I have no clue at all as to why the setTimeout( ) command is spread over three script lines. Anyway, if the above code strikes you as being unnecessarily complicated, you would be right - it can be effectively replaced by the following command:
window.setTimeout("pop.close( );", 3000);
Lightgreen is the colour
The next script line writes the pop document's body element start-tag:
pop.document.write('<body bgcolor="ltgreen">');
Above and beyond the fact that the bgcolor attribute has been deprecated for all of the elements that can/could take it, ltgreen is not a recognized color name - Joe should know and have caught this, having put together a "So, You Want A Basic Color Code, Huh?" resource for the HTML Goodies site. As you can imagine, the ltgreen value has an unpredictable effect on the actual background color of the pop document body - I'll show you how it plays out on my computer in the "In practice..." section below.
The "Basic Color Code" page does list a lightgreen color name with a corresponding #90ee90 hex code value; lightgreen is not one of the W3C's core colors, but if both Netscape/Mozilla and Microsoft give the lightgreen name a thumbs up - it appears in the "Color Values" appendix of the JavaScript 1.3 Client-Side Reference and in Microsoft's "Dynamic Color Reference" - then that's good enough for me. The background color of the document in the window in the screen shot at the beginning of the post is in fact set to lightgreen - we'll see more lightgreen backgrounds in due course.
popText
The "Hello Goodbye" script concludes with three commands that respectively write the text strings that appear in the pop window (plus a final line that closes the pop document's body element):
pop.document.write('<center><b><h2>Welcome</h2></b></center>');
pop.document.write('<center><b><h3>Please Wait... While This Page Loads</h3></b></center>');
pop.document.write('<center><b><h6>This Window Will Close Itself...</h6></b></center>');
pop.document.write('</body>'); /* As the body element end-tag is optional, this line can be commented out if desired. */
Each string is marked up as an h# element whose immediate parent is a b element. In a non-script HTML context, placing an h# element in a b element violates the content model of the b element, which can only have inline element children. Moreover, I've never come across a browser that didn't bold the h# elements by default, so I wouldn't have put those b elements in there in the first place.
Semantically, are the pop text strings really headings? The Welcome string is a heading of sorts but the other two strings definitely aren't. It would be more appropriate to mark the strings up as p elements and then vary their font sizes by setting their CSS font-size properties to x-large, large, and x-small, respectively. The p elements can be collectively bolded by a
p { font-weight: bold; }
style rule. Each string is also centered with a center element; yes, one center element start-tag (prior to the Welcome string) and one center element end-tag (following the This Window Will Close Itself... string) would have sufficed here. Better yet would be to replace the center elements with a text-align:center; style that can be applied to either the body element or the aforementioned p elements.
pop.document.write('<title>Hello Goodbye<\/title>');
pop.document.write('<style type="text/css">');
pop.document.write('body { background-color: lightgreen; }');
pop.document.write('p { font-weight: bold; text-align: center; }');
pop.document.write('#p0 { font-size: x-large; }');
pop.document.write('#p1 { font-size: large; }');
pop.document.write('#p2 { font-size: x-small; }');
pop.document.write('<\/style>');
pop.document.write('<body>');
pop.document.write('<p id="p0">Welcome<\/p>');
pop.document.write('<p id="p1">Please Wait... While This Page Loads<\/p>');
pop.document.write('<p id="p2">This Window Will Close Itself...<\/p>');
pop.document.write('<\/body>');
In practice...
ltgreen
When the bgcolor attribute of the pop document's body element is set to ltgreen, the actual background color in the pop window, as determined by the DigitalColor Meter utility, is #00ee00 when using Safari, #0000ee when using MSIE or Opera, and #000e00 when using Firefox - your guess is as good as mine as to where these color values come from:
Safari:



As indicated above, this situation can be cleared up by changing ltgreen to lightgreen; of course, you are free to choose some other shade of green or another color altogether for the pop document background.
pop features
If for whatever reason you would prefer that the Please Wait... While This Page Loads string not wrap, then increasing the pop width from 200 pixels to 325 pixels will do the trick.
Regarding the non-size features that are ostensibly set to no by the window.open( ) command (excluding menubar, which is not part of the window chrome on a Macintosh), I find that only MSIE actually disables all of them. Opera and Safari enable the resizable feature

and disable the rest of them. Firefox enables the location, status, and resizable features:

In Firefox's case, the location, status, and resizable features respectively map onto, and are overridden by, dom.disable_window_open_feature.location, dom.disable_window_open_feature.status, and dom.disable_window_open_feature.resizable browser preferences that are turned on by being set to true - according to Mozilla, this is done for accessibility and/or security reasons - these preferences can be accessed and switched to false in order to disable the corresponding window features via the Firefox about:config pane:

Demos
Like his "Quick Window" demo, Joe's "Hello Goodbye" demo, which would normally spring into action upon accessing the "Hello Goodbye" page, will not work for you if your browser is set to block pop-up windows. I recognize that pop-up blocking did not come about in order to kill
a great little welcome to those who stop by your sitethat Joe wanted to create - it's a shame the marketers had to ruin things for the rest of us, isn't it?
I should finally mention that at the end of Blog Entry #26 we very briefly discussed the "Hello Goodbye" script and provided a self-closing window demo triggered by clicking a button - let's reprise the demo here, why not?
I hope to be able to cover the next two "Opening New Windows with JavaScript" tutorials, "So, You Want A Pop-Under Window, Huh?" and "The Same Size", in the following entry.
reptile7
Saturday, July 18, 2009
How Much Is That Image in the Window?
Blog Entry #151
In the previous post, we went through HTML Goodies' "Remote Image" script in detail, but said little about how it all works out in practice - we'll do that today. Let's begin with a closer look at Joe's demo...
More on width, height, cond1, and cond2
You can use the to-be-displayed image's intrinsic width and height for the transferview( ) width and height argument values, but you don't have to. Joe himself pairs a 79px-by-114px joe2.gif image with the function's width=200 and height=300 arguments, giving the window below:

I can see why Joe did not assign 79 to width. The width of the joe2.gif image is actually smaller than that of the underlying button, which itself extends slightly beyond the right edge of the viewport for the width=100 window that is generated when width=79 is increased by 20 via the cond1 assignment statement. (A 100px-by-100px viewport is as small as you're gonna get with Firefox, MSIE, and Safari; Opera will let you take the width down to 70.)
You can see from the above image that a width=220 cond1 value for the joe2.gif image is a bit large, but not overly so, whereas a height=370 cond2 value is clearly overkill. As a sort of compromise, assigning 114 to height while keeping the width=200 argument (
onclick="transferview('/images/joe2.gif', 200, 114);"
) gives a nicely sized demo window:
The preceding screen shots were taken when using MSIE 5.2.3 for Mac OS X. In contrast, Firefox, Opera, and Safari do not place an empty line box between the joe2.gif image and the button and do not equip the height=114 window with a right scrollbar.
Centering the display
In the ImageWindow document, the joe2.gif img placeholder and the Close Window control are wrapped in a center element, which centers the display horizontally but not vertically. The center element is "shorthand" for a
<div align="center"> ... </div>
element and was deprecated by HTML 4.0. Moreover, the align attribute is now deprecated for most of the elements that can/could take it (align is still legit for the "internal" table elements - tr, td, col, etc.); align can specify a horizontal or vertical alignment for some of these elements, and only a horizontal alignment for the others - the div element is in the latter group.Should we get rid of ImageWindow's center element? We noted last time that, as CDATA, a stringified center element in a script does not violate the HTML 4.01 Strict DTD. But if we were to ever migrate the ImageWindow document to an external file in which the document is written as normal HTML, then yeah, we would want to exchange the center element for a state-of-the-art alternative. Perhaps the most straightforward way to do this is to:
(1) Replace the center element with a regular div element (as noted above, the center element is itself a type of div element).
(2) Give the div element a text-align:center; style via, e.g., (a) an inline style attribute added to the div element start-tag, (b) a style element block in the document head, or (c) a divObject.style.textAlign = "center"; JavaScript command.
Its documentation in the CSS 2.1 Specification's "Text" chapter notwithstanding, the text-align:center; style smoothly centers the joe2.gif image and button with all of the OS X browsers on my computer. The CSS text-align property applies to block-level elements, table cells, and inline-block elements, which raises the question: Why not assign the text-align:center; style to the document's body element, which is a 'root' block for all practical purposes, and then just ditch the center element? In this case, the joe2.gif img element would become a direct child of the body element, which is OK by the HTML 4.01 Transitional DTD but is forbidden by the Strict DTD, and thus not the way to go if you're setting the bar high.
Body element declaration in the Transitional DTD:
<!ELEMENT BODY O O (%flow;)* +(INS|DEL) -- document body, children can be block-level or inline -->
Body element declaration in the Strict DTD:
<!ELEMENT BODY O O (%block;|SCRIPT)+ +(INS|DEL) -- document body, children must be block-level -->
The div element has an effective width of 100%, and the text-align:center; style centers the contents of the div. Alternatively, the div element can be shrunk to 'blanket' its contents by setting its CSS width to a suitable value and then itself horizontally centered within the ImageWindow viewport à la a table element, i.e., by setting its CSS margin-left and margin-right properties to auto. For example, the button has a width of about 95 pixels (it varies slightly depending on the browser), so we might set the width of the div to 100px for centering purposes. In this case, the joe2.gif image and button will be left-justified with respect to each other (in the absence of further tweaking), but the resulting display looks fine IMO.
The form element is a block-level element, and you may be wondering, "Why don't we bring the joe2.gif image into the form that holds the button, and then apply text-align:center; to the form?" This would also be a viable option for updating the center element. Doing so will initially put the joe2.gif image and the button in the same line box; the latter can be 're-dropped' via one or two <br /> elements, per your preference.
But I would just as soon lose the form element too. The button - more precisely, its underlying input element - is a user interface element and doesn't need a form container; to put it another way, despite my use of the word "control" earlier, the Close Window input is not really a form control in the sense that we're not sending its name*/value data to a processing agent. The only practical reason to hold on to the form element is to accommodate users of ancient browsers (e.g., Netscape 4.x) that won't render form control elements outside of a form, and if you are using one of these browsers, given the ready/free availability of more recent browsers, may I kindly suggest that you consider upgrading?
*Indeed, the Close Window input doesn't even have a name attribute: FYI, this is actually a violation of both the Strict and Transitional DTDs, which require the name attribute for all input types except submit and reset - see here, e.g.
As for vertically centering the div container within the ImageWindow viewport, this can be done via the following general method:
(1) Take a screen shot of the div and obtain the div's height from the resulting image.
(2) Using the CSS position and top properties, push down the div by half of the viewport's remaining (unused) vertical space.
For example, suppose the height of the div is 140 pixels and the total height of the viewport is 370 pixels (as set by the window.open( ) command): the amount of remaining vertical space in the viewport is then 370 - 140 = 230 pixels, and thus the div can be vertically centered with a position:absolute;top:115px; style.
(A related but complementary approach to vertically centering a div element, for which we knew the height of the div but didn't know the height of the viewport, was presented in Blog Entry #140.)
Etc.
Float it
Admittedly, you may not care about centering the display; perhaps you would rather float the image left or right and then flank it with some text or whatever - I do this in my demo below.
More on transferview( )'s parameterization
The parameterization of the transferview( ) function allows it to be used with a document containing >1 images for display in ≥1 new windows. For such a document, if you feed the images' intrinsic widths to the transferview( ) width argument, then the window.open( ) strWindowName parameter, newwin + width, will cause
(a) images with the same width to be opened in a common window, and
(b) images with different widths to be opened in different windows.
If this is to your tastes, fine. If you would prefer that all of the images open in a common window, then get rid of the + width part and just set strWindowName to newwin. If you would prefer that all of the images open in different windows, then
you should use the special value _blank for strWindowName,quoting Mozilla.
We can of course expand transferview( )'s parameterization beyond the image, width, and height variables. For example,
function transferview(image, width, height, myTitle) {
...
ImageWindow.document.title = myTitle;
... }
<!-- To be triggered by: -->
onevent="transferview('myImage.gif', ###, ###, 'Title element text');"
can be used to set custom titles for a series of image windows.
More style, and a demo
At the beginning of the previous post, we noted that planting the window image in a document opens the door to styling that document and its elements per our preferences - e.g., we can give the image a custom border, we can surround the image with a custom background, etc. - I trust you can write out the CSS to do these kinds of things.
Let's wrap up our "Remote Image" discourse with a demo:
Welcome to the "Remote Image" Demo Opener Page.
A couple of comments:
• JavaScript commands are used to apply various styles to the new window's document, with one exception: an inline style attribute sets the img float property, as neither Firefox, MSIE, nor Opera would float the image JavaScriptically (although Safari would do so).
• The new window's button is "cleared" (placed below the floated image) and centered via a separate div container; like the text-align property, the CSS clear property does not apply to inline elements.
We'll take on HTML Goodies' "Hello Goodbye" script, which codes a self-closing window, in the next post.
reptile7
Wednesday, July 08, 2009
An Image Window, Revisited
Blog Entry #150
In Blog Entry #25, we showed that we can use an image URL for the strUrl parameter of the window.open( ) method
var myWindow = window.open("myPhoto.jpg", "windowName", "width=imageWidth,height=imageHeight");
and thereby display an image in a new window. HTML Goodies' "Remote Image" tutorial, our focus today, presents a script that outlines an alternate, more involved way of displaying an image in a window.open( )-opened window. In brief, the "Remote Image" script places an image in a new HTML document that is opened in a new window*, whose dimensions can be based (or not) on the intrinsic dimensions of the image. Placing the image in a parent document allows us to add some bells and whistles to the display that couldn't be added if we directly assigned the image URL to the window.open( ) strUrl parameter: we can add text and/or user interface elements (e.g., a button), we can align the image per our preference, we can impart a background color to the rest of the document body, etc.
(*We've actually done this once previously: check the newWin( ) and newWin2( ) functions in the script discussed by HTML Goodies' JavaScript Script Tips #45-48.)
The "Remote Image" script is reproduced in the div below:
<script type="text/javascript">
var width, height;
var image, ext;
var cond1, cond2;
function transferview(image, width, height) {
if (width == 0) cond1 = " ";
else cond1 = "width=" + (width + 20) + "";
if (height == 0) { cond2 = " " };
else { cond2 = "height=" + (height + 70) + "" };
var s1 = "<title>Image</title>";
var s15 = "";
var s2 = "<center><img src='" + image + "' border='0' />";
var s3 = "<form><input type='button' value='Close Window'" + " onclick='self.close( );' />";
var s4 = "</form></center>";
ImageWindow = window.open("", "newwin" + width, "toolbar=no,scrollbars=" + scroll + ",menubar=no," + cond1 + "," + cond2);
ImageWindow.document.write(s1 + s15 + s2 + s3 + s4);
ImageWindow.document.close( );
}
</script>
<form><input onclick="javascript:transferview('test.gif', 200, 300);" type="button" value="View Image" /> </form>
Deconstruction
As for the "Quick Window" script, Joe offers no deconstruction for the "Remote Image" script. Joe does provide a script demo that works with MSIE but not with Firefox, Opera, or Safari - more on this below. The demo display is accessed via a button:
<input onclick="javascript:transferview('test.gif', 200, 300);" type="button" value="View Image" />
The onclick attribute is set to a JavaScript URL; however, JavaScript URLs are meant to be paired with the href attribute of the anchor element (you can probably also pair them with the href attribute of the area element). As it is superfluous, you'd think that the javascript: part of the onclick value would throw an error and/or cancel the click action, although it doesn't (at least on my computer), but again, the javascript: doesn't need to be there and IMO should be removed. Anyway, clicking the button calls the script's transferview( ) function
var width, height, image, ext, cond1, cond2;
function transferview(image, width, height) { ... }
and passes thereto three arguments:
(0) arguments[0] (test.gif) is the file name of the image to be displayed in the new window, and is assigned to the variable image.
(1-2) arguments[1] (200) and arguments[2] (300) will be used to set the width and height of the new window, and are accordingly assigned to width and height variables, respectively.
Before moving on: as shown above, six variables, including image, width, and height, are declared globally prior to transferview( )'s declaration. The ext variable is not subsequently used in the script and should be removed from the declaration statement.
The transferview( ) function begins with a strange block of code:
if (width == 0) cond1 = " ";
else cond1 = "width=" + (width + 20) + "";
if (height == 0) { cond2 = " " };
else { cond2 = "height=" + (height + 70) + "" };
These if...else statements set values for the previously declared cond1 and cond2 variables, which will respectively become the width=imageWidth and height=imageHeight features for the window.open( ) command that opens the new window.
The if lines set cond1 or cond2 to a space character if width or height is equal to 0. Above and beyond Mozilla's assertion that the window.open( ) strWindowFeatures parameter
must not contain any blank space(this is not true in practice on a Macintosh), there's no reason why width or height should ever be 0 or set to 0 by a transferview( ) function call. If the image image were not available or if we had mistyped its file name in the transferview( ) function call, we would still be feeding non-0 numbers to width and height. If we did not specify arguments[1] and arguments[2] in the transferview( ) function call (
onclick="transferview('test.gif');"
), then in that case width and height would evaluate to undefined, not 0.The else lines are meant to set horizontal and vertical 'paddings' for the new window with respect to the image image, i.e., to push the edges of the new window away from the edges of the image. The first else line adds 20 to width, appends the sum (220) to the string width=, curiously appends to the resulting string an empty string**, and lastly assigns width=220 to cond1. In an analogous manner**, the second else line increases height by 70 and assigns height=370 to cond2.
**Perhaps the empty string operands were included to ensure that the width=220 and height=370 strings are not 'unterminated', but the Core JavaScript 1.5 Guide's "Data Type Conversion" section makes clear that this is unnecessary. Relatedly, the parentheses that surround width + 20 and height + 70 are indeed needed; otherwise, cond1 would be width=20020 and cond2 would be height=30070, i.e., the operands are concatenated in a normal left-to-right order.
Notice anything unusual about the second if line and the second else line? Both of these lines are terminated with semicolons that lie outside the if/else closing braces. Firefox, Opera, and Safari all throw syntax errors at this point in the code for the following reason: recalling that the else clause of an if...else statement is optional, these browsers view the second if line and its concluding semicolon as a complete if...else statement, and thus they expect another if clause to precede the second else clause, which cannot appear 'in isolation':
if (height == 0) { cond2 = " " };
if (false) var x = "Add me and everything is OK."; else { cond2 = "height=" + (height + 70) + "" };
There are several simple ways to solve this problem: removing the braces or the semicolon (or both) on the second if line will do it, as will the preceding code.
FYI: Mozilla briefly discusses block statements here in the Core JavaScript 1.5 Guide but says nothing about putting semicolons after them. For its part, MSIE clearly views the second if/else lines as a single if...else statement, even as the MSDN Library's "Writing JScript Code" page avers,
Notice that the primitive statements within a block end in semicolons, but the block itself does not.
In sum, there's no need to conditionalize the cond1/cond2 assignments, which can be written much more simply as:
cond1 = "width=" + (width + 20);
cond2 = "height=" + (height + 70);
We next have a series of statements that will be used to build the document that goes in the new window:
var s1 = "<title>Image</title>";
var s15 = "";
var s2 = "<center><img src='" + image + "' border='0' />";
var s3 = "<form><input type='button' value='Close Window'" + " onclick='self.close( );' />";
var s4 = "</form></center>";
Ordinarily the above strings would be plugged directly into document.write( ) commands, but separating them in this way does improve the readability of the script. It's simple enough to see what's going on here:
• The s1 string will specify a title for the new document.
• The s2 string takes care of the image image.
• The s3 string indirectly codes a button for the new window.
• Like the empty strings in the else blocks, the s15 string declaration serves no purpose and can be removed.
Some of this code - namely, the center element spanning the s2-s4 strings and the border attribute of the img element in the s2 string - would be deprecated in a non-script HTML context, and yet strings of this sort, once their </ character sequences have been escaped, will pass through a 'strict' validation because the script element has a CDATA content model.
We're finally ready to open the new window and write its document:
ImageWindow = window.open("", "newwin" + width, "toolbar=no,scrollbars=" + scroll + ",menubar=no," + cond1 + "," + cond2);
ImageWindow.document.write(s1 + s15 + s2 + s3 + s4);
ImageWindow.document.close( );
The new window's object reference will be ImageWindow and its name value will be newwin200. Via the cond1 and cond2 variables in the strWindowFeatures parameter, the ImageWindow viewport will have a width of 220 pixels and a height of 370 pixels. The strWindowFeatures parameter also sets the toolbar and menubar features to no, which is unnecessary because these features are (should be) disabled by default upon setting the width and height features.
Bizarrely, the strWindowFeatures scrollbars feature is set to the value of a scroll variable that does not appear earlier in the script - man, doesn't anyone proofread these things? I have no idea whether the script's author wanted to enable or disable the scrollbars feature, but I myself see no point in enabling it and would throw out the scrollbars/scroll code. More fundamentally, regarding the arguments passed to the transferview( ) function, width and height values for a given image should be chosen such that scrolling is not necessary: you are likely to annoy the user (it would certainly annoy me) if scrolling were needed to see the right/bottom parts of the image.
You'd think that scroll's out-of-the-blue appearance in the window.open( ) command would throw a "scroll is not defined" error: not so. Subsequent investigation has revealed that the browsers on my computer see scroll not as a variable but as a property 'reflection' of the scroll( ) method of the window object, e.g., alert(typeof scroll) displays function on the alert( ) box. As a property, scroll evaluates to function scroll() { [native code] }, which counterintuitively doesn't cause any problems vis-à-vis the strWindowFeatures string and, with respect to enabling/disabling the new window's scrollbars, is evidently converted to no by Firefox and Safari. On the other hand, with or without the scrollbars/scroll code, I find that both MSIE and Opera equip the new window with scrollbars if the new window is smaller than the displayed image.
On to the new document: the s# strings are concatenated and then written to the page via an ImageWindow.document.write( ) command; don't forget to subtract + s15 from the write( ) parameter expression if you deleted the var s15 = ""; line earlier. Per the discussion above, the new document's head has a title and its body contains an image and a button - pretty basic.
The transferview( ) function concludes with an ImageWindow.document.close( ) command, whose presence is optional, as noted in Blog Entry #148.
We'll flesh out other practical aspects of the "Remote Image" script and provide our own demo in the next entry.
reptile7
Actually, reptile7's JavaScript blog is powered by Café La Llave. ;-)