reptile7's JavaScript blog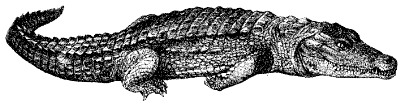
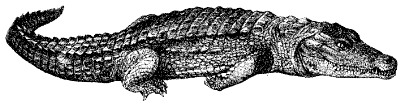
Sunday, April 24, 2005
The Primer #3 Script, Part 2
Blog Entry #6
We continue today with our discussion of HTMLGoodies' JavaScript Primers #3 and the Date object-focused script, reproduced below, that is its highlight. In our last episode, we 'microscoped' the RightNow = new Date( ); constructor statement, which allowed us to create a new Date object. With no further ado, let's get to the meat of the script, a single document.write( ) statement whose write( ) parameter contains an alternating series of (a) text-string and (b) Date_object_name.method( ) command-statement subparameters.
<SCRIPT LANGUAGE="JavaScript">
//This script posts the exact day
//and time you arrived
RightNow = new Date();
document.write("Today's date is " +
(RightNow.getMonth()+1)+
"-" + RightNow.getDate() + "-"
+ RightNow.getFullYear() + ".
You entered this Web Page at exactly: "
+ RightNow.getHours() +
":" + RightNow.getMinutes() + " and "
+ RightNow.getSeconds() +
" seconds")
</SCRIPT>
The subparameters of the write( ) parameter are connected, or concatenated, with a + sign, which functions here as a string operator. Commas can also serve as (sub)parameter delimiters; confer, for example, the document.write( ) statement in the error-laden Primer #2 assignment script, which should now look much less mysterious.
Joe cautions us not once but twice that the document.write( ) statement of the Primer #3 script "should be all on one line", and it's true that the script as typed above will throw an error, but one minor change puts things to right. In the wake of our discussion of line-breaks in JavaScript, we can quickly zero in on the problem: the ". You entered this Web Page at exactly: " text string is split between two lines. Unsplit this string, by putting it on one line, and then the script will run, in spite of its "sectional" appearance.
Three of the six command statements appearing in the write( ) parameter feature Date object methods that return date-related information: the getMonth( ), getDate( ), and getFullYear( ) methods. The getDate( ) method starts counting at 1, in an exception to the rule that JavaScript usually starts counting at 0 (starting at 0 is "a C thing", according to Webmonkey), but is otherwise straightforward, as is the getFullYear( ) method. The getMonth( ) command, however, deserves a bit of comment.
The getMonth( ) method starts counting at 0 (January), running to 11 (December), so 1 must be added to the getMonth( ) return if we want the displayed month number, written to a Web page with the document.write( ) command, to correspond to the 'normal' month number. As you might expect, addition in an arithmetic sense is also accomplished with a + sign operator, so we would write
getMonth( ) + 1
to display April, to use the current month, as 4 and not 3. Joe surrounds his getMonth( )+1 expression with a set of parentheses "so that the [arithmetic] plus sign wouldn't get confused with the [concatenation] plus signs linking everything together" - is this necessary?
Indeed it is, if we delimit the subparameters of write( ) with + signs. When I removed the outer (getMonth( )+1) parentheses and reran the script, 1 was concatenated, and not added, to the getMonth( ) return, giving "31" (and not 4) for April. On the other hand, when I replaced the concatenation + signs with commas, I found that getMonth( ) and 1 are added with or without the outer parentheses. And for the sake of completeness, I finally found that if 1 is put in quotes, converting it from a number data type to a string data type, then getMonth( ) and "1" are concatenated with or without the outer parentheses.
Three of the six command statements appearing in the write( ) parameter feature Date object methods that return time-related information: the getHours( ), getMinutes( ), and getSeconds( ) methods. The getHours( ) return follows the 24-hour clock ("military time"), starting at 0 for midnight and running to 23 for 11 PM. The getMinutes( ) and getSeconds( ) returns run from 0 to 59, similar to but not quite the same as a normal digital clock, whose first ten minute and second readings go from 00 to 09. (We'll get to a bona fide digital clock JavaScript script in Script Tips 25-28.)
As intimated by the primer, the capitalization pattern of these methods must be followed or you'll get an error. 'RightNow.getmonth( )', for example, will generate "object doesn't support this property or method" and "RightNow.getmonth is not a function" errors (once again) with MSIE and Netscape, respectively.
Quick comments on other issues
The getDay( ) method
A fourth date-related Date object method, getDay( ), gets a mention in the primer but does not appear in the script. The getDay( ) return runs from 0 (Sunday) to 6 (Saturday), as correctly stated on the HTMLGoodies JavaScript methods keyword reference page, NOT from 1 to 7, as incorrectly stated both in the primer and on the "Click Here For What You've Learned" getDay( ) page.
An interesting application of the getDay( ) method: it can be used to determine on what day of the week famous historical events of the past took place. For example:
<script language="javascript">
var date = new Date("September 17, 1787");
document.write( date.getDay( ) );
</script>
returns 1, telling us that the U.S. Constitution was signed on a Monday.
Commenting
Just before the RightNow = new Date( ); constructor statement, the primer script begins with 2 "comment" lines of text:
//This script posts the exact day
//and time you arrived
Comment lines are recognized by the browser as a non-executable part of the script - they are an 'aside' from the scriptwriter, usually (but not necessarily) relating to the script. The double slash format for commenting out single lines is discussed in the primer ("What's That // Thing?"); for commenting out >1 line, JavaScript uses a /*...*/ format that is discussed on the "Click Here For What You've Learned" Comment page and that also seems to be a C thing.
Comments do not have to be placed at the beginning of a line, but can colinearly follow a command, for example:
RightNow = new Date( ); //this is a constructor statement
It's important to emphasize that this double slash commenting business only works inside of a script. If used outside of a script, both the slashes and what follows will display on the page. The HTML <!--...--> format can be used for comments outside of a script, and is sometimes used inside of a script to comment it out for non-JavaScript-capable browsers, but I would think that just about everybody is using a JavaScript–capable browser in this day and age.
"Adding Spaces"
Joe alleges in this section that n consecutive spaces in a JavaScript text string will display as n consecutive spaces on a Web page. On my computer, however, I find that JavaScript in this situation behaves like HTML, namely, n spaces translate to 1 space. To display more than one space in a row, I have to use a series of commands, i.e., when I type:
"This is the date ", it displays as "This is the date ", but
"This is the date " displays as "This is the date ".
I'm not going to comment on the primer's concluding "A known concern:" section, which IMO unnecessarily complicates the getMonth( ) method, and a careful reading of the end-of-primer assignment and its answer didn't raise any red flags for me, so I think we'll pack it in for our discussion of Primer #3. In HTMLGoodies' JavaScript Primers #4, we'll start talking about event handlers, a JavaScript part of speech that lies at the heart of JavaScript's use in creating dynamic, user-interactive Web pages.
reptile7
Sunday, April 17, 2005
The JavaScript Date object
Blog Entry #5
Most of the JavaScript objects that we'll be dealing with in the HTMLGoodies JavaScript primers and script tips are classified as "host objects". Host objects relate, directly or indirectly, to the document containing the script and the Web browser that 'hosts' and executes the document. Host objects are somewhat browser-dependent; those host objects that are recognized by both Internet Explorer and Netscape are helpfully diagrammed, through the courtesy of Netscape, at the Web Developer's Virtual Library. In addition and to a lesser extent, we will make use of JavaScript objects known as "core objects" (also variously called "native objects", "built-in objects", "intrinsic objects", or "fundamental objects") that are not browser-dependent but are an 'intrinsic' part of the language. A couple of blog entries ago, we discussed the JavaScript document object, which is obviously a host object. The 'star of the show' in HTMLGoodies' JavaScript Primers #3, which we begin to address today, is the core JavaScript Date object, which, through several associated methods, will allow you to extract date and time information from the clock running on your computer; this information can then be posted to a Web page by use of a document.write( ) statement. Later on in the HTMLGoodies primer series, we will also see the Date object used in scripts involving random numbers, statements, and images.
For most applications, JavaScript requires the Date object to be 'defined dynamically', i.e., a new 'instance' of a Date object must be created in order to make use of the methods available to it; this is accomplished by means of a constructor statement whose general syntax is shown in the primer as:
RightNow = new Date( );
This single, simple statement offers up a smorgasbord of new concepts for us to sink our teeth into. Let's take it from left to right, shall we?
(1) Although not identified as such in the primer, RightNow is a variable name. A variable in JavaScript is very much what it is in algebra: a symbol or 'placeholder' for a particular piece of information; in this case, RightNow will symbolize a new Date object. Variables crop up briefly at the end of Primer #3 in the "A known concern:" section, and are discussed more fully in Primer #6.
Formally, a variable in JavaScript is 'declared' with the statement "var", i.e.,
var RightNow = new Date( );
however, use of the var keyword, while 'good form', is not strictly necessary.
(2) The = character marks our introduction to JavaScript operators, a key part of the JavaScript syntax. An operator in JavaScript is very much what it is in mathematics or in other technical fields: "any of various mathematical or logical processes (as addition) of deriving one expression from others according to a rule", to borrow from Webster's New Collegiate Dictionary. In our constructor statement above, = is sort of an "equals sign" but more specifically functions as an assignment operator by which the right side of the equation is 'assigned' to the left side; in this case, a new Date object is assigned to the variable RightNow.
HTMLGoodies' treatment of JavaScript operators leaves a lot to be desired, to put it politely. There's no section on operators at the HTMLGoodies JavaScript keyword reference page; similarly and no less shamefully, operators never appear on the "Commands" lists on the "Click Here For What You've Learned" pages that appear at the end of the primers. Fortunately, however, DevGuru has posted a reasonably complete page of JavaScript operators, to which we'll be referring from time to time.
(3) The new keyword is also classified as an operator; its function in creating a new JavaScript object is self-explanatory.
(4) As noted above, Date is the new JavaScript object that we are creating. As a core object, Date is always capitalized; in contrast, host objects are not capitalized. The parentheses that follow Date in the constructor statement are again good form, syntaxwise, although leaving them out will not generate an error.
(5) Finally, the constructor statement ends with a semicolon. Semicolons in JavaScript are not operators but serve as statement delimiters; they are generally "optional [but are] required if you want to put more than one statement on a single line", to quote the folks at W3Schools. (FYI: notwithstanding its name, W3Schools is not connected to the World Wide Web Consortium (W3C).)
Q & A
Q: Are there any restrictions on what a variable name can be?
A: Yes. Conveniently located in the "Naming Variables" section of the MSDN JScript Variables page are some rules to which JavaScript variable names must conform. (One exception to these rules: variable names can definitely contain dollar signs ($$$).) On this topic, Joe states, "It [the variable name for the new Date object] doesn't matter as long as the object is given an original name that isn't found in JavaScript" - he is referring here to JavaScript's reserved words, which are equivalent to the reserved words and "future reserved words" of JScript.
Q: Does the new Date object have to be 'variabilized' in the first place?
A: No. The new Date( ) constructor can be directly acted on by a relevant method, for example:
<script language="javascript">
document.write("The current year is " + new Date( ).getFullYear( ) + ".")
</script>
Output:
The current year is 2005.
Using this formulation, the set of parentheses after Date is required, or you'll get an error. Like the script in the primer, this example shows that not only plain text and HTML but also JavaScript command statements themselves can appear in the write( ) parameter.
Q: Is the "new" keyword necessary? What happens if you leave it out?
A: There are in fact a couple of Date methods that do not require the creation of a new Date object, specifically, the parse( ) and UTC( ) methods; for example:
<script language="javascript">
var ms_since_1970 = Date.parse("January 1, 2006");
document.write(ms_since_1970);
</script>
will output the number of milliseconds between January 1, 1970 and January 1, 2006:
1136091600000
In most cases, however, leaving out the new keyword will throw an error as soon as you attempt to act on Date (variabilized or not) with a method; contra the primer, a "static" Date is not created. On my computer, MSIE gives in this situation an "object doesn't support this property or method" run-time error, whereas Netscape gives a "Date_object.method( ) is not a function" error.
Q: What happens if Date is not capitalized?
A: A " 'date' is undefined" error pops up. Indeed, "date", with a lower-case "d", is an acceptable variable name for the Date object.
Q: Although Date is an object, the Date( ) syntax recalls that of the write( ) method. Does anything ever go in the parentheses that follow Date?
A: It's not necessary for the Date object to have parameters, obviously, but it can. Possible parameters for Date are listed at DevGuru's Date object page. Shown below are examples using the "dateString" and "yr_num, mo_num, day_num" parameters, respectively:
<script language="javascript">
var date = new Date("May 1, 2005");
document.write(date);
</script>
Output:
Sun May 1 00:00:00 CDT 2005
(I live in New Orleans, where it is currently Central Daylight Time.)
<script language="javascript">
var d = new Date(2005, 0, 1);
document.write( d.getDay( ) );
</script>
Output:
6
(In JavaScript, January is indexed as 0, and not 1. January 1, 2005 was a Saturday, which is indexed as 6 by the getDay( ) method.)
So much for the constructor statement. We'll address other issues of the Primer #3 script when we pick up the conversation in the next entry.
var reptile7_
Saturday, April 09, 2005
JavaScript / JScript errors
Blog Entry #4
In my first blog entry, I brazenly admitted that I do not know everything, so it is fitting that I devote some discussion to JavaScript error messages, the focus of HTMLGoodies' JavaScript Primers #2. As you might expect, a JavaScript error message announces that a mistake has been made in the writing of a JavaScript script, preventing its execution in whole or in part. Beyond THAT, however, the instructive information that is extractable from "these little jewels", as Joe Burns affectionately calls them, can vary considerably. I have typically been able to decipher JavaScript errors, and the underlying mistakes they point to, when writing my own scripts, which heretofore have been written at a relatively simple level of JavaScript. It's a different story when I encounter them while surfing the Web, however; I look at the highlighted source code of the 'offending' page and am seldom, if ever, able to figure out what the problem is. And man, do these errors pop up on the Web, all the time - there sure are a lot of sloppy 'JavaScripters' out there (including our friends at Microsoft, as we'll see below).
We kick off our discussion of JavaScript error messages by outlining how to 'access' them. Joe tells you what to do if you're a PC user; here's how I do it on my iMac:
Internet Explorer (5.1.6): Go to the Preferences command under the Edit menu. On the left-hand side of the Preferences window, select the Web Content option under the Web Browser heading. At the bottom of the Web Content settings

is an Active Content box with a group of four checkboxes, two of which relate to JavaScript: (1) the "Enable scripting" checkbox and (2) the "Show scripting error alerts" checkbox; the latter of these checkboxes, when checked, will generate a JavaScript error message window whenever MSIE encounters a JavaScript error. (Of course, the "Enable scripting" checkbox should be checked as well.) The amount of information in the error message window will vary, depending on the error, but the window does not have a "Details" button that must be clicked as is inferred by the primer.
In the absence of doing this, MSIE gives no evidence of JavaScript errors, at least on my computer. There's no "triangular sign in the lower left-hand corner" with "an exclamation point in the triangle", nor any "text proclaiming there are errors on the page", in contrast to Joe's observations vis-à-vis his PC.
Netscape (7.02): Netscape does not have an option for the automatic generation of JavaScript error message windows; instead, JavaScript errors can be accessed at the Netscape "JavaScript Console". Under the Tools menu, go to the Web Development command, and then the JavaScript Console subcommand; alternatively, and paralleling what Joe describes for Netscape in the primer, you can also launch the JavaScript Console by typing "javascript:" (no quotes) in the browser window's address/location bar and hitting Return. Outside of the JavaScript Console, however, Netscape gives no evidence (e.g., "instructions in the status bar") of JavaScript errors on my machine.
Types of Errors
Using Microsoft's 'taxonomy', Joe subdivides JavaScript errors into two types: Syntax errors and Run-time errors. (This is an MSIE thing; Netscape does recognize some errors as "syntax errors" but otherwise generally refers to them all as simply "Error[s]".) These errors reflect the two-stage process of JavaScript interpretation in which each command statement is (1) first analyzed to make sure its syntax is correct and (2) then executed. Syntax errors pertain to problems with periods, parentheses (and other punctuating enclosure marks, e.g., square brackets and braces, that will come up later), quotes, and other aspects of, well, syntax; contra the primer, misspellings are NOT syntax errors, but are recognized as run-time errors, as are incorrect capitalizations. Correspondingly, run-time errors pertain generally to command statements whose syntax is OK but which still won't execute for one reason or another.
On my computer, MSIE calls syntax errors "compilation errors". Moreover, Microsoft's reference material does suggest that JScript, the Microsoft 'knockoff' of (Netscape's) JavaScript, is compiled as well as interpreted by the Internet Explorer browser.
Since working through this primer, I have kept a running log of the different JScript errors that have popped up while surfing the Web with MSIE. Here they are*:
Microsoft JScript compilation errors
Syntax error
Expected ')'
Expected identifier or string
Unterminated string constant
Invalid character
Conditional compilation is turned off
Microsoft JScript runtime errors
Object doesn’t support this property or method
Object doesn't support this action
‘whatever’ is undefined
Object expected
Invalid procedure call or argument
Argument not optional
Permission denied
Out of memory
Out of stack space
(*All of the compilation (syntax) errors but only a couple of the runtime errors appear on the corresponding Microsoft lists linked above. Note that the "Invalid character" compilation error plagues Microsoft's MSDN Library Web sites.)
I will not attempt to define these various errors but am merely listing them here 'for the record'.
Error message information
Joe informs us, "The wonderful thing about a JavaScript error message box is that the little window that pops up tells you where and what the problem is"; this is often, but not always, true. Consider the code below containing a script whose document.write( ) statement is missing a right parenthesis:
<html>
<head>
<title>JavaScript Error Messages</title>
</head>
<body>
<script language="javascript">
document.write("Today is Thursday."
</script>
</body>
</html>
With MSIE, the script above generates an "Expected ')' " compilation error:

As you can see, the error message in this case specifies the line of the document (line 7, counting down from the top of the document, as Joe notes) and the character of that line (character 35, counting from the left with the first character indexed as 0, and not 1) that is problematic. Clicking the window's Source button opens a source-code window in which the error location is highlighted:

Unfortunately, some MSIE JScript error message windows do not give the aforementioned line and/or character information, nor a meaningful highlight in the source code - you're on your own in those cases.
Netscape is less helpful. Netscape's JavaScript Console error message for the above script is:

appears here. Netscape's description of the error, "missing ) after argument list", is similar to but less intuitive than that of MSIE. With respect to the error location, Netscape identifies line 8 as the problem, perhaps because the script will run if a right parenthesis is put at the start of line 8 (just before </script>), but I again find this less satisfying than MSIE's marking the error at line 7. In lieu of character information, Netscape points, sort of, with a little green arrow to the error, but not very accurately, as you can see. Finally, the source-code window that is opened by clicking the Source File link

has no highlight of the error.
Quick comments on other issues
I don't have anything to add to Joe's remarks (a) on margins and command lines in the "Fixing the Errors" section and (b) on propagating errors in the "Multiple Errors" section of the primer, but I do want to comment on...
"The Error Line"
This section of the primer contains some of the infamous HTMLGoodies sloppiness on which I commented in my first blog entry. Joe attempts to illustrate a truncation syntax error with some code that is initially - and wrongly, with respect to Joe's discussion of the error location - typed as 7 lines but is a little later typed rightly as 15 lines. Much more serious than this minor-but-annoying mistake is that, as written, the code in question does not in fact throw an error, but is OK. I discussed line truncation in JavaScript ad nauseam in my last blog entry, so I won't belabor the point here.
The end-of-primer assignment and its answer
Joe asks the reader to identify two errors in an 18-line block of code.
The first error, which is generated by the "...x" 3rd line, is indeed a syntax error but occurs because the line begins with a period, a no-no in JavaScript, NOT because " '...x' was not defined", as claimed in the assignment answer. (On the other hand, if the 3rd line is changed to simply "x" (without the 3 periods), then an " 'x' is undefined" run-time error does in fact pop up - try it yourself.) Joe himself notes that the " 'whatever' is undefined" error is a run-time error, as opposed to a syntax error, in the "Something's Not Defined" section of the primer.
The second error, which is generated by the "document.wrte(" ",month,"/",day,"/",year," ")" 15th line, gives an "Object doesn’t support this property or method" run-time error with MSIE and a "document.wrte is not a function" error with Netscape - the misspelling of "write" is NOT a syntax error, in accord with our discussion earlier.
And that concludes our dissection of Primer #2. HTMLGoodies' JavaScript Primers #3, which we'll hit next time, is somewhat of a 'trial by fire'; the progression in difficulty in going from Primer #2 to Primer #3 is as great, IMO, as that between any two of the HTMLGoodies JavaScript primers. If you can make it through Primer #3, then you should be able to slog your way through the rest of them.
reptile7
Friday, April 01, 2005
More on Line-Breaks
Blog Entry #3
Let's briefly revisit the subject of line-breaks in JavaScript, which I discussed in my last blog entry. In doing some 'homework' for my up-and-coming discussion of HTMLGoodies' JavaScript Primers #2, I discovered some additional, and rather surprising (to me, at least), places where line-breaks can be put in a JavaScript command statement without interrupting the execution of the script. In illustration, consider the script in the 10-line 'document' below, which features in Joe Burns's discussion in Primer #2:
<html>
<head>
<title></title>
</head>
<body>
<script language="javascript">
document.write("text for the page")
</script>
</body>
</html>
I put the above code in a SimpleText file, which I named with an ".html" extension and executed on my hard disk directly (as opposed to FTPing it with Fetch to the server space that EarthLink gives me) with either Internet Explorer (5.1.6) or Netscape (7.02). With respect to the document.write("text for the page") command statement, I found that there are no fewer than five places where one can introduce a line-break without causing any problems, specifically:
(1) between document and .write("text for the page"), i.e., just before the period;
<script language="javascript">
document
.write("text for the page")
</script>
(2) between document. and write("text for the page"), i.e., just after the period;
<script language="javascript">
document.
write("text for the page")
</script>
(3) between document.write and ("text for the page"), i.e., just before the left parenthesis (this mode of truncation appears in HTMLGoodies' JavaScript Primers #1, as you'll recall);
<script language="javascript">
document.write
("text for the page")
</script>
(4) between document.write( and "text for the page"), i.e., just after the left parenthesis;
<script language="javascript">
document.write(
"text for the page")
</script>
and (5) just before the right parenthesis (notwithstanding Joe's representation to the contrary in Primer #2);
<script language="javascript">
document.write("text for the page"
)
</script>
Line-breaks at any other location in the document.write("text for the page") line will give an error.
Moreover, extra spaces via the space bar can be added at any of the acceptable-for-a-line-break locations above, for example:
<script language="javascript">
document .write("text for the page")
</script>
(That's 10 spaces between document and .write("text for the page"), FYI.)
You can also put extra spaces between the words of the quoted code of the write( ) parameter:
<script language="javascript">
document.write("text for the page")
</script>
but you can't put line-breaks here without getting an error, as we demonstrated last time.
So there you have it - everything you wanted to know about line-breaks but were afraid to ask, at least for the time being. Practical information or academic minutiae? You be the judge. I still think it's a good idea to be cautious about where you might put line-breaks in a script, however.
reptile7
Actually, reptile7's JavaScript blog is powered by Café La Llave. ;-)