Sunday, December 11, 2011
Divising a New Content Rotator
Blog Entry #235
In today's post I'll tell you how I would code the content rotator of HTML Goodies' "Build Your Own JavaScript Content Rotator" tutorial.
The div thing
As detailed in Blog Entry #233, the original content rotator employs a table-inside-a-table approach to laying out the rotator's frame and content - I wouldn't call this a 'complicated' approach but it is more involved than it needs to be. I for my part will use a corresponding div structure to lay out the rotator.
#divOuter { width: 410px; height: 600px; background-color: black; }
#divTitle { height: 50px; text-align: center; color: #ffff66; font-weight: bold; font-size: 24pt; line-height: 1.6; }
#divContent { width: 310px; height: 500px; background-color: white; position: relative; left: 50px; }
#divCaption { font-size: 16pt; font-weight: bold; }
#slideImage { width: 300px; height: 300px; border: 1px solid; }
#divCaption, #divImage, #divDescription { position: relative; top: 50px; }
#divCaption, #divImage { text-align: center; }
#divContent, #divCaption, #divDescription { padding: 4px; }
...
<div id="divOuter">
<div id="divTitle">My Content Slide Show</div>
<div id="divContent">
<div id="divCaption">Grasslands</div>
<div id="divImage"><img id="slideImage" src="image1.jpg" alt="" /></div>
<div id="divDescription">This is some text about the Grasslands image above. This description can be as long as you want it to be.</div>
</div></div>
(1) A divOuter div handles the rotator's frame.
(2) A divTitle div contains the "My Content Slide Show" title at the top of the display. The
line-height:1.6;
style declaration serves to vertically center the title in the div (unlike vertical-align, line-height can be applied to block-level elements).(3) A divContent div plays the role of the outer frame table's central cell; it is horizontally centered in the divOuter div by a
position:relative;left:50px;
positioning.(4-6) A slide's caption, image, and description are respectively held by divCaption, divImage, and divDescription divs, which collectively are approximately centered vertically in the divContent div by a
position:relative;top:50px;
positioning*. Like the display title, the caption and image are horizontally centered in their divs by a text-align:center;
style. Lastly, the slide parts are slightly pushed apart from each other and from the frame by a padding:4px;
style.*Applying
display:table-cell;vertical-align:middle;
to the divContent div will also vertically center the caption/image/description content; however, I find that the Mozilla browsers (Firefox, Camino, Netscape 9) will not relatively position a display:table-cell;
element. Moreover, display:table-cell;
is not supported by pre-IE 8 versions of Internet Explorer.A set of six simple divs: that's all we need, folks. Ah, but what about the Tree Canopy and Mountain Clouds slides? Does the rotator HTML really need to include them? This is something I see as an accessibility issue...
Accessibility
Regarding the original code, users without JavaScript support have no access to the contentArea2 and contentArea3 slides, which is not such a big deal if those slides show photos of trees and clouds but would clearly not be a good thing if we were to use the slides for
complex layouts of product informationas suggested by the Conclusion on the tutorial's second page. Do we want all of the slides to be visible simultaneously for these users? This can be done - the contentArea2/contentArea3
display:none;
settings can be moved to a script for users with JavaScript support - and might be OK if we were dealing with smaller slides, although it would run counter to the space-saving solutionaspect of the rotator touted by the author in the tutorial's Introduction to the JavaScript Content Rotator section.
Alternatively, we could supplement the rotator with links that open the second and third slides in new windows
<p><a href="slide2.html" target="_blank">Click here to see Slide 2 in a new window.</a></p>
<p><a href="slide3.html" target="_blank">Click here to see Slide 3 in a new window.</a></p>
and if we do that, and that's what I think we should do, then there is no point in coding all three slides in the rotator HTML, whether we use a table- or div-based structure.
Script retool
If the second and third slides are externalized for users without JavaScript support, then the main document still needs to be able to access the caption/image/description data for those slides so that JavaScript-enabled browsers can generate the slides on the fly; this data can be placed in the JavaScript part of the rotator code. We can use arrays to index the data for use in the rotator loop:
var captionArray = ["Grasslands", "Tree Canopy", "Mountain Clouds"];
var imageArray = new Array(3);
for (i = 0; i < 3; i++) {
imageArray[i] = new Image( );
imageArray[i].src = "image" + (i + 1) + ".jpg"; }
var descriptionArray = ["This is some text about the Grasslands image above. This description can be as long as you want it to be.",
"This is some text about the Tree Canopy image above. This description can be as long as you want it to be.",
"This is some text about the Mountain Clouds image above. This description can be as long as you want it to be."];
In each rotator iteration,
(1) a captionArray string will be written to the divCaption div (or captionCell td, if you want to stick with a table-based layout),
(2) an imageArray image will be loaded into the slideImage img placeholder, and
(3) a descriptionArray string will be written to the divDescription div (or descriptionCell td).
These operations can be carried out automatedly via the classical animation code presented in Blog Entry #232, which lends itself very nicely to the present situation:
var slideNum = 0;
function rotateContent( ) {
slideNum++;
document.getElementById("divCaption").innerHTML = captionArray[slideNum];
document.getElementById("slideImage").src = imageArray[slideNum].src;
document.getElementById("divDescription").innerHTML = descriptionArray[slideNum];
if (slideNum == 2) slideNum = -1; }
The above rotateContent( ) function does not contain a recursive function call; per my preference, the next section's demo uses a setInterval( )/clearInterval( ) mechanism for starting and stopping the rotator.
var timer1;
window.onload = function ( ) {
timer1 = window.setInterval("rotateContent( );", changeDelay);
document.getElementById("divContent").onmouseout = function ( ) { timer1 = window.setInterval("rotateContent( );", changeDelay); }
document.getElementById("divContent").onmouseover = function ( ) { window.clearInterval(timer1); } }
Demo
The demo below is based on the code of the preceding sections.
My Content Slide Show
Grasslands
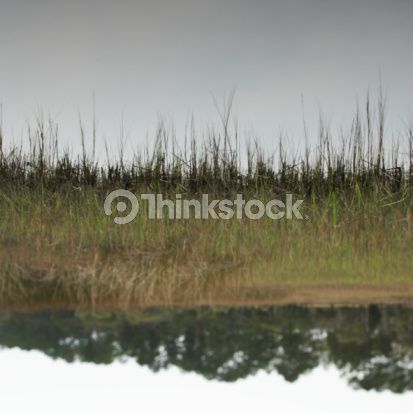
This is some text about the Grasslands image above. This description can be as long as you want it to be.
Slide 2
My Content Slide Show
Tree Canopy
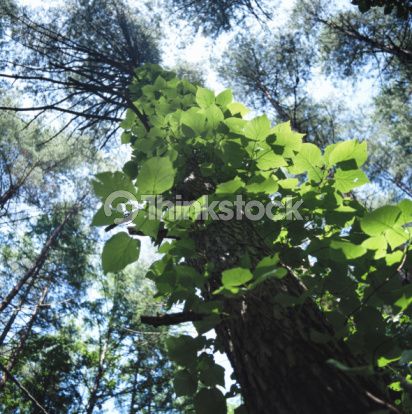
This is some text about the Tree Canopy image above. This description can be as long as you want it to be.
Slide 3
My Content Slide Show
Mountain Clouds
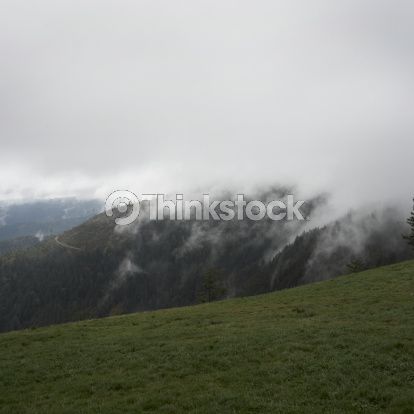
This is some text about the Mountain Clouds image above. This description can be as long as you want it to be.
Next up in the Beyond HTML : JavaScript sector is "Object Oriented JavaScript Class for Developers", which discusses what JavaScript does and does not have in common with more advanced programming languages and is not suitable for a post. The sector tutorial after that, "How To Use the JavaScript Lightbox Image Viewer", showcases an effect that is less interesting than the code that produces it, but going through that code will give us a good workout and we'll get stuck into it in the following entry.
reptile7
Actually, reptile7's JavaScript blog is powered by Café La Llave. ;-)