Thursday, March 09, 2006
Image Flipping II
Blog Entry #32
We continue our foray into image flipping as we move on to HTML Goodies' JavaScript Primers #16, "Image Flip With A Function." Consistent with its title, Primer #16 uses a JavaScript function to write the image src property. Let's get right to the Primer #16 Script (I've reshaped it and cleaned it up a bit - the center and anchor tags are not closed in the primer):
<html><head>
<title>JavaScript Example</title>
<script type="text/javascript">
function up( ) {
document.mypic.src="up.gif"; }
function down( ) {
document.mypic.src="down.gif"; }
</script></head>
<body><center>
<h2>Sample Animation</h2>
<a href="http://www.htmlgoodies.com" onMouseOver="up( );" onMouseOut="down( );">
<img src="down.gif" name="mypic" border="0">
</a></center></body></html>
As you can see, this script builds only slightly on the Primer #15 Script, in which the document.image_name.src="imagefile" commands are executed directly by the onMouseOver and onMouseOut event handlers.
Now, if the script above seems inefficient to you, well, it does to me too. If we're going to go to the trouble of functionizing the image flip commands, then can't we somehow do this with just one function? It gets worse: at the end of the "Deconstructing the Script" section of the primer, Joe discusses the extension of the Primer #16 Script to "multiple" image flips; his approach thereto is to add a new function for each new image flip command. Again, is this really necessary?
It isn't, as Joe himself seems to hint in "The Concept" of Primer #16 when he says, "For multiple JavaScript statements, call a function. In the real world, you will often need multiple JavaScript image flips on a page." And sure enough, Joe's own "So, You Want A Dual Image Flip, Huh?" tutorial, which we mentioned in the previous post and which perhaps was written later than was Primer #16, does feature an approach to executing multiple image flips with a single function. The coding in this tutorial is more complicated than it needs to be for our purposes, so we'll strip it down accordingly, as follows:
The function:
<head><title>Title text</title><script type="text/javascript">
function flip(x,y) {
document.images[x].src=y; }
</script></head>
For each image flip in the document <body>:
<img name="imagename" onmouseover="flip('imagename','imagefileB');" onmouseout="flip('imagename','imagefileA');" src="imagefileA">
(Contra Primer #16, and this is the last time I'm going to comment on this, event handlers are not case-sensitive, as we learned in Blog Entry #7.)
Let's get into some 'deconstruction' of our own, shall we? As Joe often does, we first consider the document body code, in particular, the onMouseOver and onMouseOut commands in the <img> tag, e.g.:
onmouseover="flip('imagename','imagefileB');"
A mouseover event calls a function, flip( ) - so far, so normal. Unlike function calls we've seen previously, however, the "flip( );" code in this command specifies two string parameters - 'imagename' and 'imagefileB' - that will be "passed" (i.e., sent) to the flip( ) function in the document head and assigned respectively to the x and y variable parameters in the flip( ) function declaration; in other words, calling the flip( ) function also executes the commands:
var x = 'imagename';
var y = 'imagefileB';
Now charged with values for x and y, the triggered flip( ) function plugs 'imagename' and 'imagefileB' into its image flip command statement:
document.images['imagename'].src='imagefileB';
/* although not mentioned in Blog Entry #15, we can use document.images['imagename'] to reference an <img name="imagename"> element */
which then in this case replaces the 'imagefileA' image, initially set by the <img> tag, with the 'imagefileB' image. In turn and analogously, the onMouseOut function call returns us to the 'imagefileA' image by assigning 'imagename' to x and 'imagefileA' first to y and then to document.images['imagename'].src via the flip( ) function.
Using this method, the same flip( ) function, via the x and y variables, will accept and act on the names and associated source files of other flippable images on the page. Many image flips, one function, no problem. Try it out below:
Welcome to the image flip function div.
A single onMouseOver/onMouseOut-triggered function effects each image flip below; check the current page source for the coding.
Image Flip #1:
Image Flip #2:
Image Flip #3: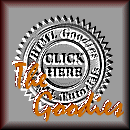
Image Flip #4:
A single onMouseOver/onMouseOut-triggered function effects each image flip below; check the current page source for the coding.
Image Flip #1:

Image Flip #2:

Image Flip #3:
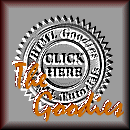
Image Flip #4:

I'm able to demonstrate image flips here at Blogger.com, but not using the function-based technique described in this entry.
Belated demo for Primer #15:
Roll your mouse over and away from the green square below to meet Vito and Sapphire (depending on your processor speed, this may take a few seconds).

The coding here follows the <img onmouseover="this.src='imagefileA';" onmouseout="this.src='imagefileB';" src="imagefileC"> format discussed in the previous post.
But actually, you might want your image flip commands to be in separate functions, which you can then customize with additional JavaScript code to your heart's content. Indeed, 'versatilizing' the image flip process that way was probably what Joe had in mind when he wrote Primer #16.
We will return to the image flip topic later in the HTML Goodies JavaScript Primers series. Meanwhile, in the next post we'll forge on to Primer #17, in which we'll see another application of a common function accepting and acting on value inputs from separate event handlers.
reptile7
Actually, reptile7's JavaScript blog is powered by Café La Llave. ;-)